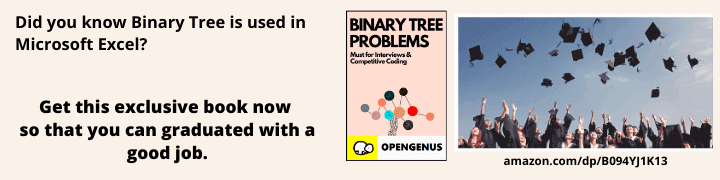
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will see in detail the need to pause a running JavaScript code and what are the different ways in which one can achieve it like SetTimeout and async/await.
Table of contents
- Why do we need to pause?
- Different ways
- Sleep
- SetTimeout
- async/await
Why do we need to pause a running code?
Let us assume you made a web page and you want to fetch data in that web page from a diffrent resource and after fetching you want to display a message called "Thank you for visiting" , so in this case fetching would take sometime because the resource is diffrent but the message to be displayed would take no time to be displayed so in order to display a message after fetching the data we need to pause this particular line of code until our data is fetched.
Example
CASE -1 WITHOUT ANY PAUSE
function hello()
{
console.log("THANKS FOR VISITING");
}
hello();
console.log("HELLO THERE");
OUTPUT
THANKS FOR VISITING
HELLO THERE
CASE-2
function hello()
{
console.log("THANKS FOR VISITING");
}
hello();
setTimeout(hello, 3000); // pausing execution of this particular line.
console.log("HELLO THERE");
OUTPUT
HELLO THERE
THANKS FOR VISITING
DIFFERENT WAYS TO PAUSE A CODE
- SLEEP
One of the method to pause the code is sleep , but javascript highly recommeds it as a bad practice because unlike other programming languages javascript is single-thread language, so if we put it on sleep it will block the thread and hence execution is terminated.
IMPLEMENTATION
function sleep(seconds) {
var currentTime = new Date().getTime();
while (currentTime + seconds >= new Date().getTime()) {
}
}
2. setTimeout()
setTimeout() method is useful when you want to execute a block of code only once but in an asynchronous manner. For example, displaying a message to a user after the specified time irrespective of the order of calling the function.
SYNTAX:
setTimeout(functionName, milliseconds);
1st parameter is name of the function
2nd parameter the time after which you want the function to be executed.
IMPLEMENTATION
console.log("Hello");
setTimeout(() => { console.log("World!"); }, 1500);
console.log("Friends");
OUTPUT
Hello
Friends
World!
3. Async/Await
Let's just talk about What is promise and than async await.
Promise is a javascript construct that represents a future unknown value. if we genralize it than one can say that it is a javascript which promises us to return something wheather be successfully fetched data or error in object , even the network error.
const promise =new Promise((resolve, reject) =>{
if(response.status == 200)
{resolve(response.body)}
else
{
const error = {...}
reject(error)
}
})
promise.then(res => {
console.log(res)
})
.catch(err =>
{console.log(err)})
So one can use promises to do asynchronous work, and can be sure that we will be getting a value in future.
Async/Await is a way of writing promises that allows us to write asynchronous code in a synchronous manner.
Implementation
const getData = async () => {
const response = await fetch("https://iq.opengenus.org/")
const data = await response.json()
console.log(data)
}
getData()
So , here also nothing has changed much from the above code as we are still using promises to fetch data, but now it looks synchronous, and we no longer have .then and .catch blocks.
Conclusion -> async/Await lets us use generators to pause the execution of a function.
With this article at OpenGenus, you must have the complete idea of DIFFERENT WAYS TO PAUSE A JAVASCRIPT CODE.