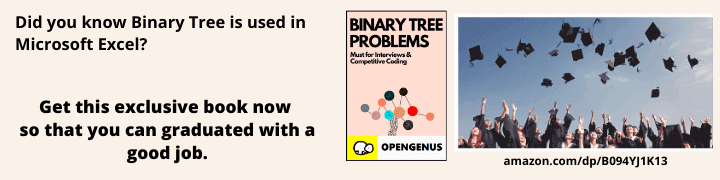
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the Random module in Python in depth and explored the different functions like seed, getrandbits, randrange, choice and much more with Python code examples.
Table of contents:
- Introduction to Random module in Python
- seed
- getrandbits
- randrange
- randint
- choice
- choices
- shuffle
- sample
- random
- uniform
- triangular
Introduction to Random module in Python
A module in python is a file with group of statements and definitions.It can contain variables, classes and functions.
random module in python is used to generate random values.
random module can be imported in our program using the following syntax
import random
If a specific function(for example, choice) needs to be imported,then the following syntax can be used
from random import choice
If all the functions are to be imported,then the following syntax can be used
from random import *
There are several in-built functions in this module.Let's go through the functions frequently used one by one.
1) seed
A seed value n is used to generate a random number sequence.
Syntax:
random.seed(n)
Setting the seed value to 5
import random
random.seed(5)
print(random.random())
0.6229016948897019
Each time the same seed value is passed the same sequence of random numbers is generated.
import random
random.seed(5)
print(random.random())
print(random.random())
random.seed(5)
print(random.random())
print(random.random())
0.6229016948897019
0.7417869892607294
0.6229016948897019
0.7417869892607294
2) getrandbits
The getrandbits function takes in number of bits n as argument and returns a random integer of n bits.
Syntax:
random.getrandbits(n)
Here the value passed is n=4.Hence,it returns a 4-bit random integer.
import random
print(random.getrandbits(4))
13
3) randrange
The randrange takes in two integers a and b as arguments and returns a random integer in-between the specified values a and b.
Syntax:
random.randrange(a,b)
Here, a is inclusive and b is exclusive meaning the interval is [a,b-1]
Taking a=2,b=9
import random
print(random.randrange(2,9))
3
4) randint
The randint function is a simple modification of randrange.
Syntax:
random.randint(a,b)
Here, a and b both are inclusive meaning the interval is [a,b]
If a,b are the input parameters
randrange(a,b) generates a random value from the interval [a,b-1]
whereas
randint(a,b) generates a random value from the interval [a,b]
Hence,randint(a,b) is same as randrange(a,b+1)
Taking a=2,b=9
import random
print(random.randint(2,9))
6
5) choice
The choice function returns a random item from a specified sequence sequence.
Syntax:
random.choice(sequence)
The sequence can be string, list or tuple.
Let a list be passed as argument.
import random
sequence=["one","two","three","four","five"]
print(random.choice(sequence))
four
Now, passing a string as argument.
import random
sequence="opengenus"
print(random.choice(sequence))
u
6) choices
The choices function takes in a sequence sequence and an integer k as arguments and returns a randomly generated sequence of size k containing values from the specified sequence sequence.
Syntax:
random.choices(sequence,k)
Passing a tuple and k=10 as arguments
import random
sequence=("google","amazon","apple")
print(random.choices(sequence,k=10))
['google', 'google', 'amazon', 'amazon', 'google', 'apple', 'apple', 'apple', 'apple', 'google']
Each item in the sequence can be assigned weights weights so that the item with highest weight has the highest probability of being chosen and so on.
Syntax:
random.choices(sequence,weights,k)
import random
sequence=("google","amazon","apple")
weights=[1,2,20]
print(random.choices(sequence,weights,k=10))
['google', 'amazon', 'google', 'apple', 'google', 'apple', 'apple', 'apple', 'apple', 'apple']
As said the element "apple" was with the highest weight and it occured the most number of times.
7) shuffle
The shuffle function takes in sequence sequence as an argument and shuffles the list in random order.
Syntax:
random.shuffle(sequence)
The shuffle function doesn't return a new list rather it modifies the exisiting list.
The sequence must be mutable(for example,list) as it will be modified.
Passing a list as argument
import random
sequence=["a","b","c","d","e","f"]
random.shuffle(sequence)
print(sequence)
['c', 'f', 'b', 'a', 'e', 'd']
8) sample
The sample function takes a sequence sequence and an integer k as arguments and returns list of size k containing items randomly chosen from the specified sequence.
Syntax:
random.sample(sequence,k)
k must be less than or equal to the size of the specified sequence
import random
sequence=[1,2,3,4,5]
print(random.sample(sequence,k=4))
[3, 2, 1, 5]
9) random
The random function generates a random floating point number between 0 and 1.
Syntax:
random.random()
Here 0 is inclusive and 1 is exclusive meaning the interval is [0.0,1.0)
import random
print(random.random())
0.28979005625006504
10) uniform
The uniform function takes in two integers a and b as parameters and returns a random floating point number in between a and b.
Syntax:
random.uniform(a,b)
Here, a and b both are inclusive meaning the interval is [a,b]
import random
print(random.uniform(25,70))
35.014086669240854
11) triangular
The triangular function takes in two integers a and b as parameters and returns a random floating point number in between a and b.
Syntax:
random.triangular(a,b)
Here, a and b both are inclusive meaning the interval is [a,b]
import random
print(random.triangular(7,77))
39.2308642640529
The above case works similar to uniform.
In addition, a mode parameter can be set.The mode lets us generate a random floating point number close to one of the given parameter values.
Syntax:
random.triangular(a,b,mode)
By default the mode value is the midpoint of the specified parameters.Hence,the probability of number being generated is not favoured for any parameter.
import random
print(random.triangular(6,93,10))
9.420306033285689
It can be seen that 6,93 were passed as arguments and mode value is set to 10.The floating point number generated is close to 6.
With this article at OpenGenus, you must have the complete idea of Random module in Python.