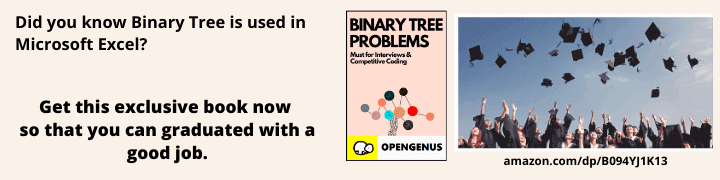
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we have explored how we can round and truncate numbers in C using the math.h library.
math.h is a header file in the list of standard C libraries which enables the coder to perform some basic mathematical operations and transformations. The header file contains many functions among which some commonly used functions are pow(), ceil(), floor(), sqrt(), trunc(), round() and abs(). Most of the functions in math.h uses floating point numbers.
In the following article we will be discussing about the two familiar functions:
- round(): roundl(), lround(), lroundl(), lroundf()
- trunc(): truncl(), truncf()
To use round() and trunc(), you need to import the header file:
#include <math.h>
round()
-
round() function in c is used to return the nearest integer value of the float/double/long double argument passed to this function.
-
If the decimal value is from ".1 to .5", it returns an integer value which is less than the argument we passed and if the decimal value is from ".6 to .9", the function returns an integer value greater than the argument we passed. In short the round() function returns an integer value nearest to the argument we passed.
-
There are two types of rounding functions in math.h library.
1. round()
It takes a floating value as argument and rounds the decimal part of it. It returns value in floating type format.
If we pass float number as an argument, the syntax is:
roundf (float arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("roundf(+2.311) gives = %+.1f\n", roundf(2.311));
//here %+.1f is used to print only one place after decimal
}
Output:
roundf(+2.311) gives = +2.0
If we pass double as an argument, the syntax is:
round (double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("round(+2.64) gives = %+.1f\n", round(2.64));
//here %+.1f is used to print only one place after decimal
}
Output:
round(+2.64) gives = +3.0
- If we pass long double as an argument, the syntax is:
roundl (long double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("roundl(+2.72) gives = %+.1f\n", roundl(2.72));
//here %+.1f is used to print only one place after decimal
}
Output:
roundl(+2.72) gives = +3.0
2. lround()
- It also takes a floating value as argument and rounds the decimal part of it, similar to round(). The only difference is lround() returns a value in integer type format.
- If we pass float number as an argument, the syntax is:
lroundf (float arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("lroundf(+2.72) gives = %d\n", lroundf(2.72));
}
Output:
lroundf(+2.72) gives = +3
- If we pass double as an argument, the syntax is:
lround (double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("lround(+5.81) gives = %d\n", lround(5.81));
}
Output:
lround(+5.81) gives = +6
- If we pass long double as an argument, the syntax is:
lroundl (long double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
printf("lroundl(+2.64) gives = %d\n", lroundl(2.64));
}
Output:
lroundl(+2.64) gives = +3
Note
- If arg is ±∞, it is returned, unmodified
If arg is ±0, it is returned, unmodified - If arg has type long double, roundl, lroundl, llroundl is called. Otherwise, if arg has integer type or the type double, round, lround, llround is called. Otherwise, roundf, lroundf, llroundf is called, respectively.
trunc()
- The trunc() function allows to truncate(remove) the decimal value from a floating number and return an inetger value.
- There are three types of truncate functions in math.h library
1. trunc()
-
It takes a double value as argument and truncates the decimal part of it. It returns a double value whose decimal value is zero(0).
-
Syntax:
double trunc(double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
double x1 = 3.0, x2 = 3.9, x3 = -3.3, x4 = -8.9;
printf("Truncated value of %.1f is %.lf \n",x1,trunc(x1));
//here %.1f is used to print only one place after decimal
printf("Truncated value of %.1f is %.lf \n",x2,trunc(x2));
// For negative values
printf("Truncated value of %.1f is %.lf \n",x3,trunc(x3));
printf("Truncated value of %.1f is %.lf \n",x4,trunc(x4));
return 0;
}
Output:
Truncated value of 3.0 is 3
Truncated value of 3.9 is 3
Truncated value of -3.3 is -3
Truncated value of -8.9 is -8
2. truncf()
- It works same as trunc() function but with the difference that it is used for float values instead of double. It takes a float value as argument, removes digits after decimal point and return modified float value.
- Syntax:
float truncf(float arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
float x1 = 3.1, x2 = 3.91, x3 = -3.3, x4 = -8.9;
printf("Truncated value of %.1f is %.lf \n",x1,truncf(x1));
//here %.1f is used to print only one place after decimal
printf("Truncated value of %.1f is %.lf \n",x2,truncf(x2));
// For negative values
printf("Truncated value of %.1f is %.lf \n",x3,truncf(x3) );
printf("Truncated value of %.1f is %.lf \n",x4,truncf(x4) );
return 0;
}
Output:
Truncated value of 3.11 is 3
Truncated value of 3.91 is 3
Truncated value of -3.3 is -3
Truncated value of -8.9 is -8
3. truncl()
- It works same as trunc() and truncf function but with the difference that it is used for long double values instead of double and float. It truncates the values after decimal and returns the modified value.
- Syntax:
long double truncl(long double arg);
Example:
#include <stdio.h>
#include <math.h>
int main()
{
long double x1 = 3.04, x2 = 3.98, x3 = -3.31, x4 = -8.9;
printf("Truncated value of %Lf is %Lf \n",x1,truncl(x1) );
//here %Lf is format specifier used for long double
printf("Truncated value of %Lf is %Lf \n",x2,truncl(x2) );
// For negative values
printf("Truncated value of %Lf is %Lf \n",x3,truncl(x3) );
printf("Truncated value of %Lf is %Lf \n",x4,truncl(x4) );
return 0;
}
Output:
Truncated value of 3.04 is 3
Truncated value of 3.98 is 3
Truncated value of -3.31 is -3
Truncated value of -8.9 is -8
Remember
round() is used to round a floating number to its nearest integer value whereas trunc() is used to remove the decimal values from a number.
With this, you have the complete knowledge of rounding and truncating a number in C.