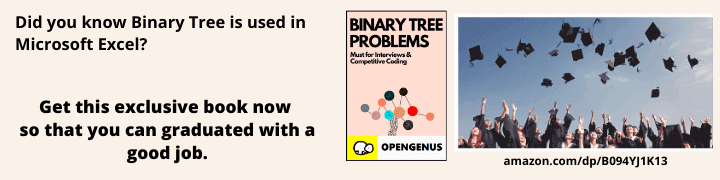
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we have explored how to post a text as a tweet in Twitter using Twitter API in Python. We have used to approaches:
- Using tweepy library
- Using Requests library for HTTP requests
Before we go into the approach, we will go through some basic information about Twitter, API and characteristics of Twitter API.
Twitter is an online news and social networking site where people communicate in short messages called tweets. We can use Python for posting the tweets without even opening the website. There is a Python library which is used for accessing the Python API, known as tweepy. Here, we are going to use tweepy for doing the same.
What is an API (Application Program Interface)?
- An application program interface (API) is a set of routines, protocols, and tools for building software applications. Basically, an API specifies how software components should interact.It is a gateway that lets us access a server's internal functionality, in our case twitter
- API is the way for an application to interact with certain system/application/library/etc. For example, there are API's for OS (WinAPI), API's for other applications (like databases) and for specific libraries (for example, image processing), etc. APIs are usually developed in a form consumable by a client application
Steps to perform the task
- Register for Twitter API and get your keys
- Install dependencies
- Write our script
Twitter API
- The Twitter API is simply a set of URLs that take parameters. They URLs let you access many features of Twitter, such as posting a tweet or finding tweets that contain a word, etc.
- Twitter allows you to interact with its data tweetsand several attributes about tweets using twitter API.
- Twitter API's can be accessed only via authenticated requests.
characteristics of Twitter API
- The twitter API uses JSON data format for returning and receiving the data.
- The twitter API is HTTP-based (over SSL) API meaning we can use get method to retrieve data from twitter,post method to send requests to the twitter server and search method to search the twitter posts.
- The twitter API limits the number of requests that can be sent to the twitter server per access token or twitter account.This is called twitter rate limit.If you encounter twitter rate limit exceeded error it means that Twitter rejected consecutive attempts to access its API under your Twitter account.The rate limit is different for different methods of the API.
- The methods of twitter API accepts various parameters which are used to cusotmize the requests according to needs.
- There are twitter API libraries for almost all programming languages.
Read the Documentation of twitter API from here
Getting Twitter API keys
To start with, we will need to have a Twitter developer account and obtain credentials (i.e. API key, API secret, Access token and Access token secret) on the to access the Twitter API, following these steps:
- Create a Twitter developer account https://developer.twitter.com/
- Go to https://developer.twitter.com/en/apps and log in with your Twitter user account.
- Click “Create an app”
- Fill out the form, and click “Create”
- A pop up window will appear for reviewing Developer Terms. Click the “Create” button again.
- In the next page, click on “Keys and Access Tokens” tab, and copy your “API key” and “API secret” from the Consumer API keys section.
- Scroll down to Access token & access token secret section and click “Create”. Then copy your “Access token” and “Access token secret.
Approach 1: Tweepy
What is tweepy?
Tweepy is a python dependency that we will be using to generate tweets.Tweepy is a Python library for accessing the Twitter API. It is great for simple automation and creating twitter bots. Tweepy has many features.
Read the Documentation of tweepy from here
Install tweepy using command:
pip install tweepy
Python Code and Explanation
After importing the dependencies, first we want to create 4 variables that will authenticate with Twitter.You will find all the required variables in your
developer's account dashboard and we can copy and paste each of them as strings.
#importing all dependencies
import numpy as np
import tweepy
#variables for accessing twitter API
consumer_key='XXXXXXXXXXXXXXXXXXXXXXXXXX'
consumer_secret_key='XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
access_token='XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
access_token_secret='XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
Now, we'll create a variable called off for auth and use the OAuth handler method of tweepy. This method takes two arguments the consumer key and the consumer secret. This method was written inside of the twepy library and all of its functionality is in our hands.Now we have to call the set access_token method on the auth variable which takes two arguments the access token and the access token secret and that's it we've created our authentication variable now.
#authenticating to access the twitter API
auth=tweepy.OAuthHandler(consumer_key,consumer_secret_key)
auth.set_access_token(access_token,access_token_secret)
api=tweepy.API(auth)
Now that we have our API variable we can perform many different possible tasks.
We consider 2 cases-
In first case, make a simple textual tweet using update_status method which takes the tweet string as input.
In the second, case we make a textual tweet with attached image media using update status with media method which takes image_path and a string as input.
tweet=input('enter the tweet')
#Generate text tweet
api.update_status(tweet)
tweet_text=input('enter the tweet ')
image_path =input('enter the path of the image ')
#Generate text tweet with media (image)
status = api.update_with_media(image_path, tweet_text)
api.update_status(tweet_media)
Approach 2: Requests
What is Requests package
The requests module allows you to send HTTP requests using Python.
The HTTP request returns a Response Object with all the response data (content, encoding, status, etc).
We will be using the post method of requests library to send a request to tweet.
Install requests using command:
pip install requests
Python Code And Explanation
After importing the dependencies, first we want to create variables(consumer_key and consumer_secret) that will authenticate with Twitter.You will find all the required variables in your developer's account dashboard and we can copy and paste each of them as strings.
#importing all dependencies
import numpy as np
import tweepy
import requests
import base64
#Define your keys from the developer portal
consumer_key = 'XXXXXXXXXXXXXXXXXXXXXX'
consumer_secret = 'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
The twitter API requires a single key that is a string of a base64 encoded version of the two keys separated by a colon so we will encode the consumer keys into base64 which is the usable form.
#Reformat the keys and encode them
key_secret = '{}:{}'.format(consumer_key, consumer_secret_key).encode('ascii')
# Transform from bytes to bytes that can be printed
b64_encoded_key = base64.b64encode(key_secret)
#Transform from bytes back into Unicode
b64_encoded_key = b64_encoded_key.decode('ascii')
Now, We will use requests package of python to post an authentication request using twitter authentication resource URL to the twitter server and store the post response in a variable. We To check and make sure that the request worked , We will print the status code of the request response. If the status code printed is 200 then the request worked successfully.
base_url = 'https://api.twitter.com/'
auth_url = '{}oauth2/token'.format(base_url)
auth_headers = {
'Authorization': 'Basic {}'.format(b64_encoded_key),
'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8'
}
auth_data = {
'grant_type': 'client_credentials'
}
auth_resp = requests.post(auth_url, headers=auth_headers, data=auth_data)
print(auth_resp.status_code)
access_token = auth_resp.json()['access_token']
Output:
Now, we will assign a dictionary variable with the parameter we want to pass into the post request. Here, the status key of dictionary variable is assigned with 'Hello World' which is the message we want to tweet.After this we will assign a variable with the resource URL where we will be sending the request to the twitter server.Now use the post method of requests package to send the tweet request and store the response into a variable.
Get different resource URL's from here
post_params = {
'status': 'Hello World',
}
post_headers = {
'Authorization': 'Bearer {}'.format(access_token)
}
post_url = 'https://api.twitter.com/1.1/statuses/update.json'
post_resp = requests.post(post_url,headers=post_headers,params=post_params)
Output:
Tweet posted
With this, you have the complete knowledge of posting a tweet in Twitter using the Twitter API. Enjoy.