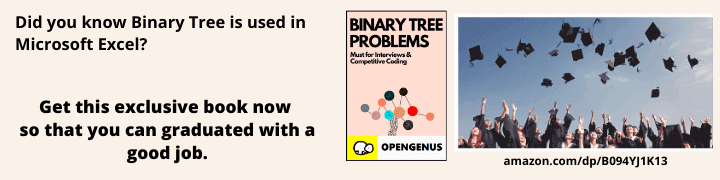
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Static and extern are storage classes in C which defines scope and life-time of a variable. Similar to any variables in C, we can use these keywords with pointers for different use cases.
Table of content:
- Static pointers in C/ C++
- Extern pointers in C/ C++
Let us get started.
Static pointers in C/ C++
Static variables once initialized exists until the termination of the program. These variables holds their value even after they are out of their scope. Once declared and initialized they are not re-declared i.e., once the memory is allocated, then they exist till the termination of that program. A static pointer declaration as shown below.
static int * ptr; // A static pointer of type int is defined
This declaration says that the pointer itself should have static storage duration. and does not say anything about the storage class of the thing the pointer points to. That could change at different times during program's execution simply by assigning different values to this pointer.
Scope of these variables depends on where they are declared. Their scope is local if the variable is declared inside the function. If static variable is declared in globle region then it can be accesses anywhere in the program or in that perticular C file. A initial value 0 or NULL is assigned by the compiler.An example for the same is given below.
#include <stdio.h>
void foo(){
int a = 10, b = 20;
static int *c; //static pointer variable declaration
//re-declaration is not done in case of static variables
if(c == 0)
c = &a;
else
c = &b;
printf("value = %d\n", *c);
printf("address of pointer = %d\n", &c);
printf("address of memory pointed by pointer c = %d\n", c);
printf("address of a = %d\n", &a);
printf("address of b = %d\n", &b);
}
int main(){
foo();
foo();
}
Output:
value = 10
address of pointer = 4218932
address of memory pointed by pointer c = 6422204
address of a = 6422204
address of b = 6422200
value = 20
address of pointer = 4218932
address of memory pointed by pointer c = 6422200
address of a = 6422204
address of b = 6422200
In the above program a function foo() is called from main(). In first iteration static variable as initialized by compiler contains '0'(zero), so value of 'a' will be assigned and as it is static variable it holds this even after completion of function execution. So that in second iteration of foo() static pointer 'c' is assigned to b's value. We can observe the same in the output window. Address of static pointer is constant throughout the execution of program. But where it points-to can be modified.
A static pointer can be used to implement a function that always returns the same buffer to the program. This can be helpful in serial communication.
char * Buffer(){
static char *buff;
if(buff == 0)
buff = malloc( BUFFER-SIZE );
return buff;
}
Extern pointers in C/ C++
Extern storage class specifies that the variable is defined elsewhere in a large program. By specifying a variable as Extern, programmer can use a variable that is declared elsewhere as if it is locally declared. Extern variable is nothing but a global variable initialized with a legal value where it is declared in order to be used elsewhere. These variables can only be initialized globally and only once, but they can be declared any number of times as per requirement. The variables declared as extern are not allocated any memory.
#include <stdio.h>
void foo(){
int a = 11;
extern int *ptr;
//specifies compiler to search for this variable outside this function
printf("Default value = %d (NULL pointrer)\n", ptr);
ptr = &a;
printf("After assignment %d\n", *ptr);
}
int main(){
foo();
}
int *ptr; // Globle pointer declaration
// pointer initialized by compiler - null pointer
Output:
Default value = 0 (NULL pointrer)
After assignment 11
By using Exrern pointers globally available pointers can be created and can be useful is different scenarios. One of its use is accessing command line arguements from main function in other files or function of a project.
extern int ArgC = 0;
extern char ** ArgV = 0;
int main( int argc, char ** argv ) {
ArgC = argc;
ArgV = argv;
...
}
With this article at OpenGenus, you must have the complete idea of Static and extern pointers in C/ C++.