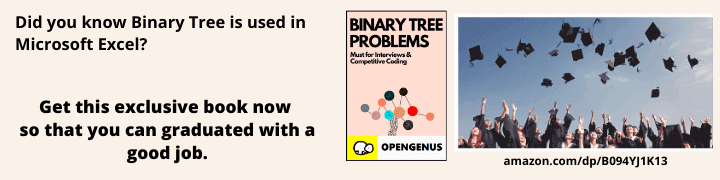
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will discuss about the concept of capturing and bubbling in JavaScript (Event Listeners) in depth with code demonstration.
Table of contents:
- Event Bubbling vs Event Capturing
- Bubbling
- Capturing
- Demonstration of Capturing and Bubbling
Event Bubbling vs Event Capturing
There are two ways of event propagation in the HTML DOM:
- Bubbling
- Capturing
Bubbling
In bubbling the inner most element's event is handled first and then the outer most element.
Capturing
In capturing the outer most element's event is handled first and then the inner most element.
Basically in simple words Bubbling is from (Bottom to Up) and Capturing is from
(Up to Bottom).
And now to understand it more clearly we will take some examples and understand how exactly the concept of Bubbling and Capturing works .
Syntax :-
addEventListener(event, function, useCapture);
Example :-
document.getElementById("myP").addEventListener("click", myFunction, true);
Here we can see that the thrid parameter is true that means we are using the method of capturing that means basically the (Top to Bottom approach).
document.getElementById("myDiv").addEventListener("click", myFunction, false);
Here we can see that the thrid parameter is falsr that means we are using the method of bubbling that means basically the (Bottom to Top appraoch) and if the third parameter is not written as true or false , then it is automaticaLly considered as false that means we are using by default the Bubbling method.
Demonstration of Capturing and Bubbling
Following is the Demonstration of Capturing and Bubbling:
JavaScript addEventListener()
Figure 1
Bubbling:
Click me!
Figure 2
Capturing:
Click me!
In Figure 1 we can see that on clicking on the bubbling we will get You clicked the bubbling element and if we click on the click me we will get You clicked the click element and then You will also get You clicked the bubbling element .
So here we can understand that on clicking the particular element then it display the message from that element and goes to the top until it has reached the end.
Code for Figure 1:
<style>
#myDiv1 {
background-color: coral;
padding: 50px;
}
#myP1 {
background-color: white;
font-size: 20px;
border: 1px solid;
padding: 20px;
}
</style>
<meta content="text/html; charset=utf-8" http-equiv="Content-Type">
</head>
<body>
<h2>JavaScript addEventListener()</h2>
<h2>Figure 1</h2><br>
<div id="myDiv1">
<h2>Bubbling:</h2>
<p id="myP1">Click me!</p>
</div>
<script>
document.getElementById("myP1").addEventListener("click", function() {
alert("You clicked the click element!");
}, false);
document.getElementById("myDiv1").addEventListener("click", function() {
alert("You clicked the bubbling element!");
}, false);
In Figure 2 we can see that on clicking on the capturing we will get You clicked the capturing element and then You will also get You clicked the click element and if we click on the click me we will get You clicked the click element.
So here we can understand that on clicking the particular element then it display the message from that element and goes to the bottom until it has reached the down.
Code for Figure 2:
<style>
#myDiv2 {
background-color: coral;
padding: 50px;
}
#myP2 {
background-color: white;
font-size: 20px;
border: 1px solid;
padding: 20px;
}
</style>
<meta content="text/html; charset=utf-8" http-equiv="Content-Type">
</head>
<body>
<h2>JavaScript addEventListener()</h2>
<h2>Figure 2</h2><br>
<div id="myDiv2">
<h2>Capturing:</h2>
<p id="myP2">Click me!</p>
</div>
<script>
document.getElementById("myP2").addEventListener("click", function() {
alert("You clicked the click element!");
}, true);
document.getElementById("myDiv2").addEventListener("click", function() {
alert("You clicked the capturing element!");
}, true);
</script>
With this article at OpenGenus, you must have the complete idea of Capturing and Bubbling in JavaScript (Event Listeners).