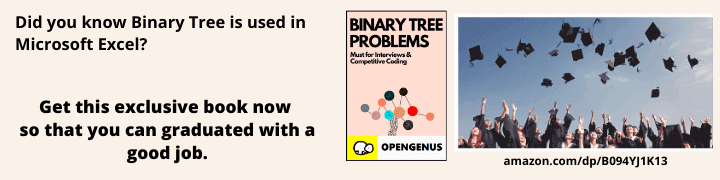
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
pwd (Present Working Directory) command is one of the basic bash line command used for getting the path of the directory we are currently working on! The command pwd itself abbreviates to "present working directory". We will use the headerfile dirent.h for directory structures and objects and implement our pwd command in C/C++. This will involve the getcwd() function call. So lets move on to the initial setup:
Initial Setup
Make a directory named "command" and inside that make a directory named "include", also in the include/ directory place the header file "dirent.h".
Also make a file "pwd.cpp" where our main implementation code will reside.
Implementation
So the basic idea is to use the getcwd
function inbuilt in the unistd.h
First we will include the header files:
-stdio.h
: Used for defining FILENAME_MAX
and cout/cin
functions.
-dirent.h
: Used for handling directory objects and pointers.
-unistd.h
: Used for importing various pre-defined variables such as _POSIX_VERSION
, _POSIX2_VERSION
,etc.
Now we also need to define or rename some functions such as _getcwd
and getcwd
to GetCurrentDir
.
So after the including header files, it'll look like,
#include <stdio.h> /* defines FILENAME_MAX */
// #define WINDOWS /* uncomment this line to use it for windows.*/
#ifdef WINDOWS
#include <direct.h>
#define GetCurrentDir _getcwd
#else
#include <unistd.h>
#define GetCurrentDir getcwd
#endif
#include<iostream>
Now after including the header files, we will implement the function to get the current working directory,
std::string GetCurrentWorkingDir(void){
//Logic here
}
We will define a character array with FILENAME_MAX
size,
char buff[FILENAME_MAX];
Now we will call the function GetCurrentDir()
to get the path of the current working directory,
GetCurrentDir( buff, FILENAME_MAX );
After this, we'll assign the path to another string as to return it from the function,
std::string current_working_dir(buff);
Lastly, we'll return the string obtained,
return current_working_dir;
So the final function implementation would look like,
std::string GetCurrentWorkingDir( void ) {
char buff[FILENAME_MAX];
GetCurrentDir( buff, FILENAME_MAX );
std::string current_working_dir(buff);
return current_working_dir;
}
As we have to call this function, we will do it from main()
function of the code,
int main(){
std::cout << GetCurrentWorkingDir() << std::endl;
return 1;
}
Final working code
#include <stdio.h> /* defines FILENAME_MAX */
// #define WINDOWS /* uncomment this line to use it for windows.*/
#ifdef WINDOWS
#include <direct.h>
#define GetCurrentDir _getcwd
#else
#include <unistd.h>
#define GetCurrentDir getcwd
#endif
#include<iostream>
std::string GetCurrentWorkingDir( void ) {
char buff[FILENAME_MAX];
GetCurrentDir( buff, FILENAME_MAX );
std::string current_working_dir(buff);
return current_working_dir;
}
int main(){
std::cout << GetCurrentWorkingDir() << std::endl;
return 1;
}
Running steps
For running the command we will first compile it using make
command in Linux, and then run it using ./pwd
,
As the command is giving output of the path /home/sahil/Desktop/command
which is the current directory we are working on, our implementation is successful.