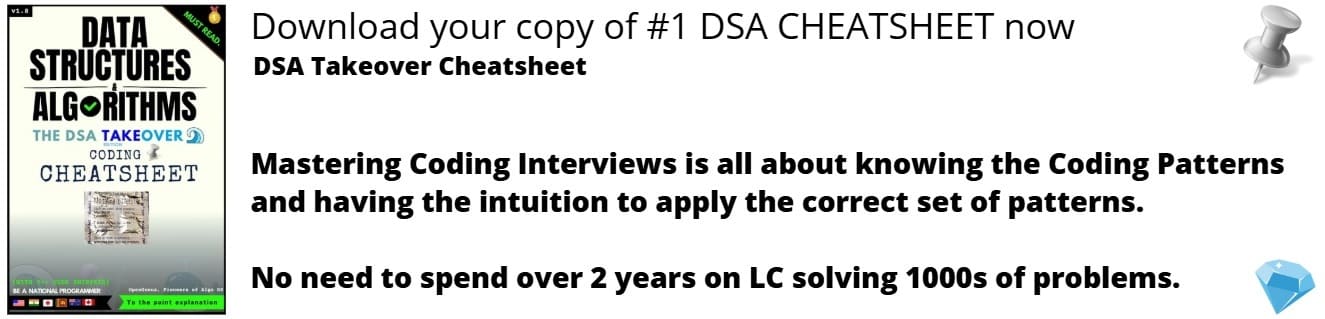
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The return value of main() function shows how the program exited. The normal exit of program is represented by zero return value. If the code has errors, fault etc., it will be terminated by non-zero value.
Table of contents:
- main() in C / C++
- The exit() function
main() in C / C++
Here is the syntax of main()
function in C/C++ language,
int main() {
// Your program
return 0;
}
Â
The valid C/C++ main signatures are:
int main()
int main(int argc, char* argv[])
int main(int argc, char** argv)
Â
In C/C++ language, the main()
function can be left without return value. By default, it will return zero.
It is prohibited to write void main()
by the C++ standard which when written result in this compitation error:
prog.cpp:4:11: error: '::main' must return 'int'
void main()
^
Â
Some compilers allow the usage of void main()
. It 'works' because the compiler does its best to generate code for programs. Compilers such as GCC will warn about non-standard forms for main()
, but will process them. The linker isn't too worried about the return type; it simply needs a symbol main
and when it finds it, links it into the executable. The start-up code assumes that main has been defined in the standard manner. If main() returns to the startup code, it collects the returned value as if the function returned an int, but that value is likely to be garbage. So, it sort of seems to work as long as you don't look for the exit status of your program.
So what happend if main is defined in the following manners?
auto main() -> int {}
auto main() { return 0; }
auto main() {}
In (1) It is legal and the program will execute successfully.
In (2) and (3), the return type of the main function cannot be deduced since the standard documentation will be reworded as:
An implementation shall not predefine the main function. This function shall not be overloaded. It shall have a declared return type of type int, but otherwise its type is implementation-defined.
The exit() function
Â
This function terminates the calling process immediately.
The declaration of this function is void exit(int status)
where status is the status value returned to the parent process.
Example:
#include <stdio.h>
#include <stdlib.h>
int main () {
printf("Start of the program....\n");
printf("Exiting the program....\n");
exit(0);
printf("End of the program....\n");
return(0);
}
Â
The result of this program will be:
Start of the program....
Exiting the program....
Â
Then what is the difference between exit()
and return
?
return
returns from the current function; it's a language keyword likefor
orbreak
.exit()
terminates the whole program, wherever you call it from.
Here is an example that could demonstrate this point:
Â
Example with return
:
#include <stdio.h>
void f(){
printf("Executing f\n");
return;
}
int main(){
f();
printf("Back from f\n");
}
Â
The output will be:
Executing f
Back from f
Â
Same Example with exit()
:
#include <stdio.h>
#include <stdlib.h>
void f(){
printf("Executing f\n");
exit(0);
}
int main(){
f();
printf("Back from f\n");
}
Â
The output will be:
Executing f
Back from f
With this article at OpenGenus, you must have a strong idea of Return value of main() in C / C++.