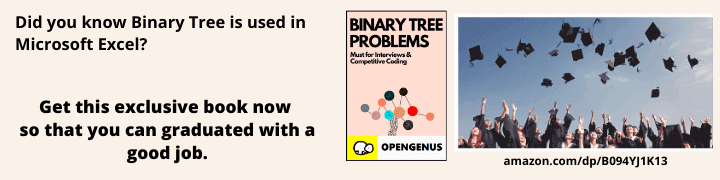
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Lets begin by defining a node.js module, Node.js module is basically a collection of javascript functions and objects that are used by external applications. However, any node.js file can be considered as a module if its data is made available to external applications. Modules enables us to share our code to other developers, they enables us to make applications that are scaled to complexity. Modules can be reused in many applications even larger applications. Creating a module is an essential skill for a Node.js developer.
Let us learn by creating a module by simply taking a string as an argument and printing it in upper case letters.
For this, you will need to install node.js and npm in your development environment. for installing it on macOS refer to this article -> Install on macOS For installing it on ubuntu follow this -> Install on Ubuntu NPM will be installed once node.js is installed on the system.
Before starting, you must be familiar with package.json file. For becoming familiar follow this article -> How To Use Node.js Modules with package.json
So, lets begin with our application step by step ->
Creating Module
We will use the node.js built-in "exports" property to make the function available to other applications. This module will contain a function and a string argument.
Every time the user calls the function with some string as a parameter, the functions returns the string as an Upper Case String.
Therefore, make a new folder called "modules". You can name the folder whatever you want.
mkdir modules
cd modules
After moving into the folder run ->
npm init
Output for the above command will be ->
{
"name": "modules",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
Now, create a new file called "index.js" and open it in any editor of your choice.
After opening index.js, we will create the logic of code in this file. So, write the following code ->
var exports=module.exports={};
Here, the "exports" keyword is used so that the function can be used by other applications. "Exports" keyword is used to reference a global object that is available in every node.js module. All functions and objects stored in exports object are called when other node.js modules import it.
Now, write the following code ->
exports.upperCase=function(name) {
return name.toUpperCase;
};
Here, we created a function called "upperCase" (You can name your function whatever you want) that takes an argument and converts it into upper case.
Now, we have created our module for printing the user provided string letter into upper case letter. Its time to call this module by other application.
Importing your local module
It is always better to import modules by their names so that the import is not broken when the context is changed. In this step, we will install our module with npm’s local module install feature.
Firstly, setup the new module outside our "modules" folder. Move out of the "modules" folder and make a new directory ->
mkdir otherApp
cd otherApp
Now, initialize your folder with npm ->
npm init
The following package.json will be generated ->
{
"name": "otherApp",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Now install your original module named "modules" with the following command ->
npm install --save ../modules
We just installed your "modules" module in the new project. Open the package.json file to see the new local dependency ->
package.json file ->
{
"name": "otherApp",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"NodeModules": "file:../modules"
}
}
Now, our "modules" module is copied to "node_modules" directory.
Now in the "otherApp" directory, make another "index.js" file. Our program will first import the "modules" module. It will then take the argument of the function and finally, it will print the letter given by the user in upper case.
Enter the following code in index.js ->
const text = require('text');
console.log(text.upperCase('opengenus'));
In our case, for example, I have passed the argument as "opengenus".
Now, run the file with the following command ->
node index.js
Output ->
OPENGENUS
Now, we have successfully installed "modules" module and can use in any other application.
Lets head towards publishing your own module ->
Publishing Modules
We can also publish our own modules in our github repository. From this, we can directly install our module in any system by using npm install command.
To publish our module, follow the below given steps ->
- First, create a repository on your github page.
- Now, we need to tell npm the author of the module and email id of the author. All this details will be added to the module when it is published. Follow the commands fo setting the author name and email ->
npm set init.author.name "xyz"
npm set init.author.email "email@email.com"
You can add the author name and email of the author by your own.
- Now, login into npm. To logging in use the following command ->
npm login
- Now, initialize your package by creating package.json file ->
npm init
- Now, comes the publishing of the module to your github repository. To publish it follow the below commands ->
git add .
git commit -m "your-commit-message"
git push origin master
- Now, final step is to publish your module. To do this, Follow the command below
npm publish
Now, with this article at OpenGenus, your module is created and published and ready to be used by other node.js applications. Enjoy.
Learn more:
- Introduction to Modules in Node.js by Priyanka Yadav
- Introduction to Node.js by Priyanka Yadav
- Downloading server files in Node.JS application by Prateek Sharma
- Creating a Very Simple Web API in Node.JS by Priyanka Yadav
- List of Node.JS topics at OpenGenus