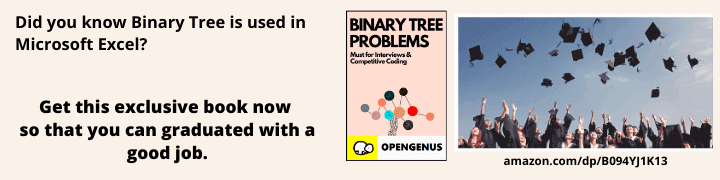
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Now that's a catchy title, but I promise you'll get it by the end of this post or not 😉 I can't promsie😊
What is a promise?
Why or When do we make a promise?
Promises in JavaScript are somewthat similar to the promises that we make in real-world. We generally make a promise as an act of insurance or sureity to the other person that what we tell will happen or we are going to make it happen/work.
Suppose I made a promise to my math teacher that I'm gonna solve a math problem as my homework assignment:
Example
let solveProblem = new Promise(function(resolve,reject))
{
//me trying to solve the problem
//usually code here contains, what we are supposed to do
let solve=true;
//true if I solved it and false if I failed to solve the problem
//Teacher checking my status below
if(solve)
{
//What next if I solve it
resolve('Solved');
}
else
{
//Teacher makes this move in-case I haven't solved the problem
reject('Not Solved');
}
});
Explanation
The above code is very simple, first we are declaring a promise with the name solveProblem and Promise
is the keyword used for promises in JavaScript, and the callback funtion has two arguments:
- resolve: represents fulfillment or meaning that the promise is sucessful i.e you did finish what you said you would.
- reject: is the action to be taken when the promise returns false meaning the promise is unfinished.
Next Steps
solveProblem.then(function(solvedProblem){
//if promise is fullfilled then do this
console.log('Congratultions! Problem'+solvedProblem);
}).catch(function(notSolved){
//if promise fails then do this
console.log('Try again, problem'+notSolved);
})
Explanation
Here consider the above code as continuation, now after finding out what happened to the promise we have to do so action in response to the outcome hence we use the keyword then
to call a function, when the promise is sucessful else catch
to call a funtion when the promise fails.
And notice the arguments in both the funtions solvedProblem and notSolved these argumnets are passed from resolve and reject in their respective cases.
Summing up everything if the problem is solved and solve returns true next if executes resolve and then calls the function and we get the output:
Congratulations! Problem Solved
else:
Try again, problem Not Solved.
But why use promises?
So now we got a basic understanding of promises, this can be also be done even with 'EventListener' functions,but why use them?
The reason behind using promises is, as we know that javascript is a single threaded language meaning that only one script can run at a time, even if you had to run mutiple tasks at a time they can only be qeued and execute one after the other. And there are certain cases where we need to check before-hand weather a certain task is completed or not and react to it based on the outcome like loading not a single image but a set/collection of images.
This can happen in asynchronous tasks and failure events, in that scenario we can use promises with then as a callback function to after the event is sucessful or a fail, so the appropriate callback is called based on the outcome of the event.
Advantages of Promises
- Multiple callbacks are possible by adding then as many times as we need them
- Promises can be chained, i.e one then of a promise can call the then of another promise which is very helpful when we want to check the happening of several events, like a chained reaction.
States of a promise
A promise is unsually in one of these theree states:
- pending: the initial state, the promise is neither sucessful nor a failure
- fulfilled: represents that the promise if sucessful
- rejected: represents that the promise in not fulfilled.
Thoughts
Promises are unlike final callbacks, they are fast and react on what needs to be done when an event is either sucessful or a un-fulfilled.
But a single promise can resolve or reject only once and that is final. Immediate resolve or reject can be called in the promise itself this prevents and avoid any kinds of errors before they even occur. And promises represent events that are already happening.