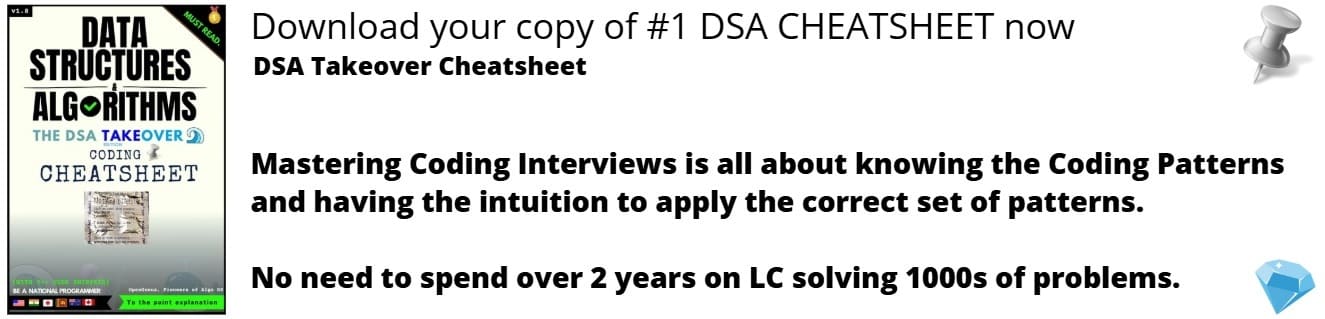
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered the differences between 3 core terms Definition, Declaration and Initialization in C and C++ along with code snippets.
Table of contents:
- Declaration
- Definition
- Initialization
- Conclusion / Table of Differences
To understand the difference between the two we should first understand each term independently.So,here we go.
1. Declaration
Declaration of a variable is generally a introduction to a new memory allocated to something that we may call with some name.
Properties of declaration -
1.Memory creation occurs at the time of declaration itself.
2.Variables may have garbage values.
3.Variables cannot be used before declaration.
Synatx-
//declare a variable
int x
//declare a function but not defined
void func();
Code-
#include<iostream>
using namespace std;
int main()
{
int x; // variable declaration
cout << "Value of x:" << x << endl; // garbage value
cout << "Address of x: " << &var << endl; // x's assigned address
cout << "Size of x: " << sizeof(x) <<" bytes";// allocated memory in bytes
return 0;
}
Output-
Value of x:32766
Address of x: 0x7ffebb87a084
Size of x: 4 bytes
2. Definition
In declaration, user defines the previously declared variable.
Syntax-
//declaration
int x;
float y;
// definition
x = 1;
y = 78;
Code-
#include<iostream>
using namespace std;
int main()
{
int x; //variable declaration
cout << "Value of x: " << x << endl; // garbage value
x = 2; // variable initialization/definition
cout << "Value of x:" << x << endl;
x = 4 + x; //data overriding
cout << "New x value: " << x << endl;
return 0;
}
Output-
Value of x: 0
Value of x: 2
New x value: 6
3. Initialisation
Initialisation is nothing but assigning the value at the time of declaration.
syntax-
int x = 0;
Code-
#include<iostream>
using namespace std;
int main()
{
// declaration & initialization at same time
int x = 1;
float x2 = 1.25;
cout << "Value of x: " << x << endl;
cout << "Value of x2: " << x2 << endl;
return 0;
}
Output-
Value of x: 1
Value of x2: 1.25
Conclusion / Table of Differences
From the above explanation we can conclude the following-
- Declaration is just naming the variable.
- Definition does not means declaration '+' Initialisation as definition might be without initialisation.
- Initialisation is assigning valueto the declared variable. (At the time of declaration)
Declaration | Definition | Initialisation |
---|---|---|
1.Declaration is just naming the variable. | Definition is declarartion without intialisation. | initialisation is declaration with definition at thesame time. |
2.Variables may have garbage values | Variables may or may not have garbage values | Variables do not have garbage values |
3 Declaration can be done any number of times. | Definition done only once | Initialisation done only once |
4.Memory will not be allocated during declaration | Memory will be allocated during definition | Memory will be allocated during initialisation |
5.Declaration provides basic attributes of a variable/function. | definition provides details of that variable/function. | Initialisation provides details of that variable/function and value. |
With this article at OpenGenus, you must have the complete idea of Definition vs Declaration vs Initialization in C/ C++.