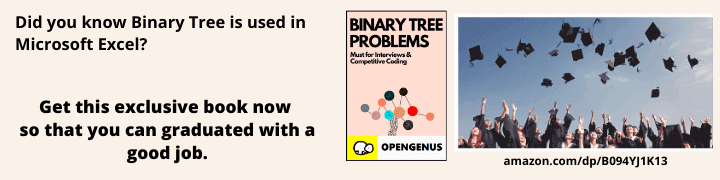
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
HTML DOM (Document Object Model) is the structure of HTML elements that defines the way a web page is displayed on a browser. HTML DOM consists of various elements, such as headings, paragraphs, tables, and images, among others.When creating a website, it's common to use HTML elements to structure and style the content on a page. However, sometimes, it may be necessary to delete or remove an element from HTML DOM. Deleting an element from HTML DOM can be accomplished in a variety of ways, depending on the task at hand. In this article, we will explore different ways to delete an element in HTML DOM.
There are three ways to delete an element in HTML DOM:
- Method 1: removeChild()
- Method 2: remove()
- Method 3: innerHTML
Method 1: removeChild()
The first method to remove an element from HTML DOM is to use the removeChild() method. This method removes a specified child node of the specified element.
Here is an example displaying the removeChild() method:
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="myDiv">
<p>This is a paragraph.</p>
<button onclick="removeElement()">Remove Paragraph</button>
</div>
<script>
function removeElement() {
var parent = document.getElementById("myDiv");
var child = document.querySelector("p");
parent.removeChild(child);
}
</script>
</body>
</html>
In the above example, we have a div element with an id of "myDiv" that contains a paragraph element and a button element. When the button is clicked, the removeElement() function is called. This function uses the removeChild() method to remove the paragraph element from the div.
Method 2: remove()
The second method to remove an element from HTML DOM is to use the remove() method. This method is similar to the removeChild() method but can be called directly on the element to be removed.
Below code uses the remove() method:
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="myDiv">
<p>This is a paragraph.</p>
<button onclick="removeElement()">Remove Paragraph</button>
</div>
<script>
function removeElement() {
var child = document.querySelector("p");
child.remove();
}
</script>
</body>
</html>
In the above example, we have a div element with an id of "myDiv" that contains a paragraph element and a button element. When the button is clicked, the removeElement() function is called. This function uses the remove() method to remove the paragraph element from the DOM directly.
Method 3: innerHTML
A third way to remove an element from HTML DOM is to use the innerHTML property. The inerHTML property sets or returns the HTML content of an element. By setting the innerHTML property to an empty string, we can delete the content of an element.
Below code shows how innerHTML property is used to delete elements:
<!DOCTYPE html>
<html>
<head>
<title>Remove Element Example</title>
</head>
<body>
<div id="myDiv">
<p>This is a paragraph.</p>
<button onclick="removeElement()">Remove Paragraph</button>
</div>
<script>
function removeElement() {
var child = document.querySelector("p");
child.innerHTML = "";
}
</script>
</body>
</html>
In above example, we have a div element with an id of "myDiv" that contains a paragraph element and a button element. When the button is clicked, the removeElement() function is called. This function uses the innerHTML property to set the content of the paragraph element to an empty string, effectively deleting the paragraph from the DOM.
Conclusion
There are several ways to delete an element from HTML DOM, and each method has its own advantages and disadvantages. The removeChild() method and the remove() method are similar in that they both directly remove the element from the DOM. However, the remove() method is more concise and can be called directly on the element itself. On the other hand, the removeChild() method may be more useful if you need to remove a specific child node of an element.
The innerHTML property is a different approach to deleting an element from the DOM. Instead of removing the element itself, it deletes the content of the element. This method may be useful if you want to keep the element itself but remove its content is not required.
It is important to note that when removing an element from HTML DOM, any event listeners or data associated with the element may also be removed. Therefore, it is a good programming practice to consider the impact of removing an element before doing so.
Moreover, it is equally important to consider the performance implications of removing the elements from HTML DOM. Removing an element from the DOM can be a computationally expensive operation, especially if the element has a lot of child nodes or if it is deeply nested within the DOM hierarchy. In some cases, it may be more efficient to simply hide the element instead of removing it from the DOM entirely. This can be achieved by setting the CSS display property of the element to "none" using JavaScript or CSS.
Below is an example:
<!DOCTYPE html>
<html>
<head>
<title>Hide Element Example</title>
<style>
.hidden {
display: none;
}
</style>
</head>
<body>
<div id="myDiv">
<p>This is a paragraph.</p>
<button onclick="hideElement()">Hide Paragraph</button>
</div>
<script>
function hideElement() {
var child = document.querySelector("p");
child.classList.add("hidden");
}
</script>
</body>
</html>
In the above example, we have a div element with an id of "myDiv" that contains a paragraph element and a button element. When the button is clicked, the hideElement() function is called. This function adds the "hidden" class to the paragraph element using the classList property. The "hidden" class sets the display property of the element to "none", effectively hiding it from view an not deleting it from DOM hierarchy.
With this article at OpenGenus, you must have the complete idea of how to delete an element in HTML DOM.
In conclusion, deleting elements from HTML DOM is a common operation when creating websites, and there are different ways to delete an element from HTML DOM. The removeChild() method, the remove() method, and the innerHTML property are all useful tools for removing elements from the DOM, and each has its own advantages and disadvantages. Above and over that, it is equally important to consider the performance implications of removing elements and to evaluate whether it is more appropriate to simply hide the element instead. With these tools at your disposal, you can improve the functionality and user experience of your web pages.