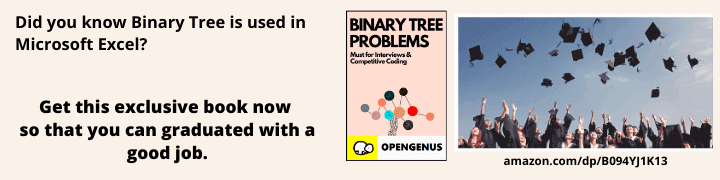
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
As a web developer, it's important to know when a user changes tabs or minimizes the browser window. This information can be useful for analytics, user engagement, and other purposes. However, traditional JavaScript event listeners are not sufficient for detecting tab changes, which can be a challenge for developers. In this article, we will discuss how to use the Page Visibility API to detect tab changes in HTML and JavaScript.
Solution
The Page Visibility API provides an event that fires whenever the user switches tabs or minimizes the browser window. This API can be used to track the number of times a user switches tabs and the duration of each tab session.
Implementation
To implement the Page Visibility API, you need to add an event listener for the visibilitychange event to the document object. When this event fires, you can check the value of the document.hidden property to see if the page is currently visible or hidden. If the page is hidden, you can calculate the duration of the current tab session by subtracting the start time (which is saved in a variable) from the current time. If the page becomes visible again, you can increment a tabCount variable and reset the startTime variable.
Here is an example code snippet that demonstrates how to use the Page Visibility API to track tab changes and session duration:
let tabCount =0;
let startTime =0;
let duration = 0;
const tabCountElement = document.getElementById("tab-count");
const durationElement = document.getElementById("tab-duration");
// Listen for the visibilitychange event
document.addEventListener("visibilitychange", function(){
if(document.hidden){
// Tab switched or minimized
duration += Date.now() - startTime;
}
else{
// Tab active again
tabCount++;
startTime = Date.now();
}
tabCountElement.textContent = tabCount;
durationElement.textContent = duration;
})
// Listen for the beforeunload event to record final duration
window.addEventListener("beforeunload", function() {
duration += Date.now() - startTime;
tabCountElement.textContent = tabCount;
durationElement.textContent = duration;
});
In the above code, we use the getElementById() method to get references to the HTML elements where we want to display the tab count and duration. We then use the textContent property to set the text content of these elements to the updated values of the tabCount and duration variables whenever the visibility of the page changes or the beforeunload event fires.
To display the tab count and duration on the HTML page, we need to add two elements to our HTML code, like so:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Detect tab change</title>
<script src="./index.js"></script>
</head>
<body>
<h1>Detect Tab Change</h1>
<p>Tab switched <span id="tab-count">0</span> times for a total duration of <span id="tab-duration">0</span> ms.</p>
</body>
</html>
Here, we have added two span elements with id attributes of "tab-count" and "tab-duration". These are the elements where we will display the tab count and duration values respectively.
Limitations
Although the Page Visibility API is a useful tool for detecting tab changes, it has some limitations. For example, not all browsers support this API, and there may be performance issues when using it on large websites or pages with many elements. Additionally, the API may not work correctly in certain scenarios, such as when the user has multiple tabs open or when using certain browser extensions.
Demo
To see this code in action, you can copy and paste it into your own HTML and JavaScript files and open the HTML file in a web browser. Then, try switching between tabs and see how the console logs the number of tab switches and the duration of each session. You can also modify the code to suit your own needs and experiment with different features of the Page Visibility API.
Conclusion
Detecting tab changes is an important aspect of web development, and the Page Visibility API provides a useful solution to this problem. By using this API, you can track the number of times a user switches tabs and the duration of each tab session, which can provide valuable insights into user behavior and engagement. However, it is important to note that this API is not supported by all browsers and may not work in some older versions. Therefore, it is important to consider fallback options or alternative solutions for detecting tab changes to ensure compatibility across different browsers and devices. Overall, the Page Visibility API is a powerful tool that can enhance the user experience and provide valuable data for website optimization and analysis.