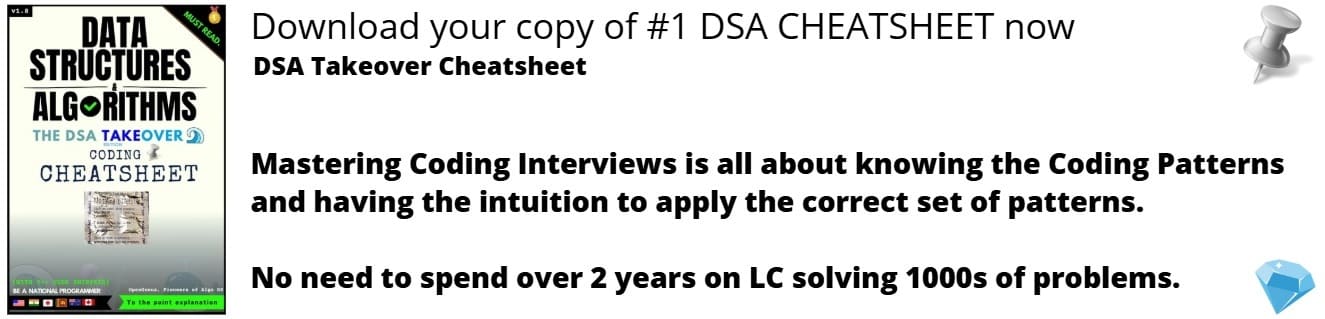
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
In today's world, we tend to open multiple tabs while working or browsing the internet. Often we may accidentally open the same URL in multiple tabs, which can lead to confusion and slow down our browsing experience. Therefore, it is important to be able to detect whether the same URL is already open in another tab. In this article, we will explore how to check if the same URL is open in another tab.
Problem statement
The problem arises when we open multiple tabs and accidentally open the same URL again. This can cause confusion and slow down our browsing experience. It is important to be able to detect whether the same URL is already open in another tab to avoid such confusion.
Solution
We can use the window.open() method to open a new tab and assign a unique identifier to that tab. We can then check whether the same URL is open in another tab by iterating through all the tabs and checking if the URL matches the current URL. If a match is found, we can focus on that tab and close the newly opened tab. If no match is found, we can keep the newly opened tab open.
Sub-sections:
- Opening a new tab with a unique identifier
- Checking whether the same URL is open in another tab
- Closing the newly opened tab
- Keeping the newly opened tab open
Opening a new tab with a unique identifier:
To open a new tab with a unique identifier, we can use the window.open() method and assign a unique identifier to the tab as follows:
var myWindow = window.open("", "myTab_" + Date.now());
This will open a new tab with a unique identifier in the format myTab_<timestamp>
.
Checking whether the same URL is open in another tab:
To check whether the same URL is open in another tab, we can iterate through all the tabs and check if the URL matches the current URL as follows:
function checkForDuplicateTab() {
var currentUrl = window.location.href;
var tabs = window.opener ? window.opener.window.frames : window.frames;
for (var i = 0; i < tabs.length; i++) {
try {
var tabUrl = tabs[i].location.href;
if (tabUrl === currentUrl) {
tabs[i].focus();
window.close();
return true;
}
} catch (e) {}
}
return false;
}
Closing the newly opened tab
If a match is found, we can focus on that tab and close the newly opened tab as follows:
tabs[i].focus();
window.close();
Keeping the newly opened tab open:
If no match is found, we can keep the newly opened tab open as follows:
return false;
Demo:
Here is a demo of the solution:
<!DOCTYPE html>
<head>
<title>Check for duplicate tab</title>
<script>
function checkForDuplicateTab() {
var currentUrl = window.location.href;
var tabs = window.opener ? window.opener.window.frames : window.frames;
for (var i = 0; i < tabs.length; i++) {
try {
var tabURL = tabs[i].location.href;
if (tabURL === currentUrl) {
tabs[i].focus();
window.close();
return true;
}
} catch (e) {}
}
return false;
}
function openNewTab() {
var myWindow = window.open("", "myTab_" + Date.now());
if (!checkForDuplicateTab()) {
myWindow.location.href = "https://iq.opengenus.org";
}
}
</script>
</head>
<body>
<button onclick="openNewTab()">Open new tab</button>
</body>
</html>
In this demo, we have a button that opens a new tab using the openNewTab() function. If the same URL is already open in another tab, the newly opened tab will be closed and the focus will be shifted to the already open tab. If the URL is not already open in another tab, the newly opened tab will be directed to https://iq.opengenus.org. Note: This demo only checks for duplicate tabs within the same browser window. If you have multiple browser windows open, it may not detect tabs that are open in a different window.
Conclusion
In conclusion of the article at OpenGenus, checking whether the same URL is open in another tab can help avoid confusion and speed up our browsing experience. We can use the window.open() method to open a new tab with a unique identifier and then check whether the same URL is open in another tab by iterating through all the tabs and checking if the URL matches the current URL. If a match is found, we can focus on that tab and close the newly opened tab. If no match is found, we can keep the newly opened tab open.