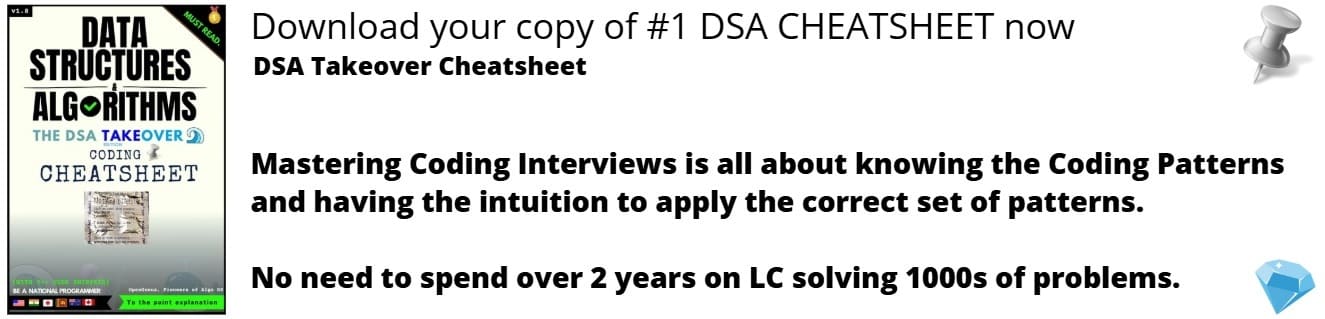
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
So this day we are going to implement one of the great topic in C/C++ programming language, and that is the "cd" command. The term "cd" stands for "Changing directory" of the filesystem, it has been implemented in the shell several years back. Also this command remains one of the most famous and notable commands in the UNIX. So basic command yet so powerful and necessary.
We will move towards the implementation of this command, we could implement this with the help of "dirent" repository available on github, you could download it here: github.com/tronkko/dirent
Initial Setup
Make a directory named "opengenus" also make a file "cd.cpp" where our main implementation code will reside. We will write our code in this cpp
file itself.
Now we would proceed to the implementation of the command,
Implementation
So we would use the function chdir
for changing the directory, the chdir
function is the default function present in the C++ library and can be used for various directory functions. On some systems, this command is used as an alias for the shell command cd. chdir changes the current working directory of the calling process to the directory specified in path.
The basic syntax of the function is:
int chdir(const char *path)
This function is defined in the unistd.h
header file and can be used for directory handling purposes. Here the parameter path
is to where we want to change the directory and go to. We would implement the functionality as, first including the header files necessary for the function which are:
- unistd.h
- stdio.h
//Importing necessary header files
#include<stdio.h>
#include<unistd.h>
After this the function has to be implemented,
int main(){
char s[100];
//Printing the current working directory
printf("%s\n",getcwd(s,100));
//Changing the current working directory to the previous directory
chdir("..");
//Printing the now current working directory
printf("%s\n",getcwd(s,100));
return 0;
}
Note : Basic idea on getcwd and chdir
The function getcwd
works but pointing to the current directory we are working on, it has the implementation in C which corresponds to obtaining the absolute path of the file we are working on with the help of directory pointers.
Also the function chdir()
has similar implementation in C but the only diffrence is that it takes a path on the filesystem and points to the given argument path. This in turn changes the current working directory.
After combining it in a single file it'll look like this,
So after the implementation part, we would run the required file by using the make
command first to compile it and then ./cd
to run the file.
$ make cd
Now after building the file we would use ./cd
to run the file,
$ ./cd
After running the file, we could cleary see the printed output and the directory is changing as well,
So the implementation is completed with the desired output. Here the user could also change the directory to any valid path he/she wants to go.