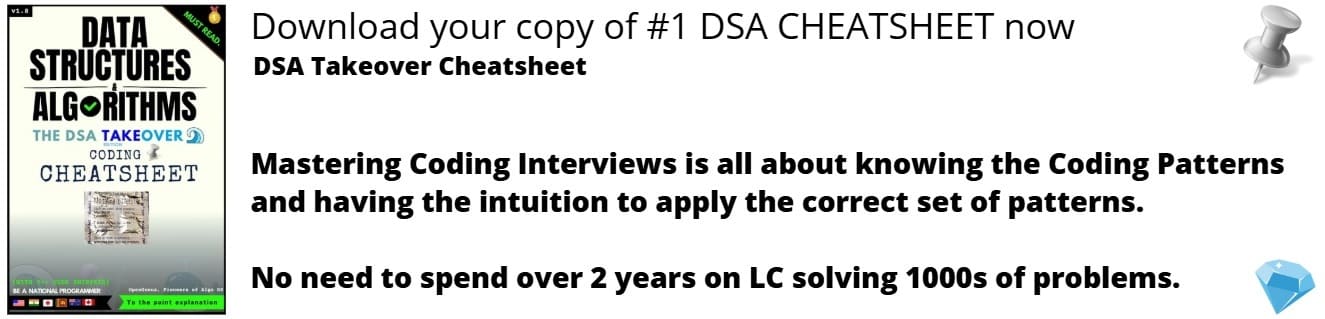
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we test our Python Knowledge by going through 50 Multiple Choice Questions.
1. Python is a ____ programming language?
(a) Compiled
(b) Object-oriented
(c) Interpreted
(d) Scripting
Ans: (b), (c), (d)
Explanation: We can employ an Object-oriented model of prgramming using Python classes and objects. We can also write scripts. Python is NOT a compiled language rather it is an interpreted language meaning that it is executed directly without any compilation using a python interpreter. On Linux, we can open the python interpreter by writing;
$ python
2. What case do Python keywords use?
(a) lowercase
(b) uppercase
(c) capitalize
(d) Both capitalize and uppercase
Ans: None of the above
Explanation: In python keywords such as 'True' and 'False' and 'None' are capitalized while the rest such as 'def', 'return', and 'float' are in lowercase.
3. Which among the following will define a block of code in Python?
(a) Brackets
(b) 'def' keyword
(c) Indentation
(d)'class' keyword
Ans: (c)
Explanation: In Python a block of code is defined using indentation, these are the whitespaces at the beginning of a line. Brackets are NOT used in Python, and neither are semi-colons. The 'def' keyword is used to define functions in Python. The 'class' keyword creates a new class with attributes.
4. Which among the following is NOT a Python keyword?
(a) True
(b) true
(c) and
(d) or
Ans:(b)
Explanation: In Python 'True', 'False' and 'None' are the only keywords that are in uppercase the rest are in lowercase. The 'and' keyword determines if both left and right operands are truthy or falsy. The 'or' keyword determines if at least one of the operands is truthy.
5. Select an order in which Python looks for an identifier.
(a) built-in namespace -> global namespace -> local namespace
(b) local namespace -> global namespace -> built-in namespace
(c) built-in namespace -> local namespace -> global namespace
(d) local namespace -> built-in namespace -> global namespace
Ans: (b)
Explanation: Python looks for an identifier by first searching the local namespace followed by the global and finally the built-in namespace.
6. Which among the following parses options received from the command line?
(a) getarg
(b) getopt
(c) main
(d) os
Ans: (b)
Explanation: The getopt is a command-line parser based on the Unix getopt() function. 'main' is the starting point in most python programs. os is a python module for interacting with the operating system.
7. Which of the arithmetic operators is used with Python strings?
(a) +
(b) *
(c) %
(d) /
Ans: (a), (b)
Explanation: We can add and multiply strings using + and * respectively.
8. Which among the following can be used to create an empty set?
(a) ()
(b) {}
(c) set()
(d) new set()
Ans: (c)
Explanation: We create new sets in Python by using the set() function. We can create new dictionaries using '{}'.
9. Which among the following correctly represents a Python tuple?
(a) [1, 2, 3]
(b) {1, 2, 3}
(c) (1, 2, 3)
(d) {1, b, 3}
Ans: (c)
Explanation: We use round brackets to store Python tuple items. Python lists use square brackets, they can be compared to arrays in other programming languages such as Java.
10. Which among the following is NOT a Python keyword?
(a) finally
(b) for
(c) from
(d) global
Ans: None of the above.
Explanation: The 'finally' keyword is used when specifying code that should execute no matter what occurs in the try-except or else blocks. The 'for' keyword is used for looping. The 'from' keyword is used when we need to import python packages. The 'global' keyword is used when we want to modify a variable defined in the global scope.
11. What is the type of 'inf' in Python?
(a) Boolean
(b) String
(c) Complex
(d) Float
Ans: (d)
Explanation: Infinity is a special floating point number. We can get inf by writing; float('inf').
12. Which among the following has the highest precedence in the expression?
(a) Exponential
(b) Addition
(c) Parenthesis
(d) Multiplication
Ans: (c)
Explanation: The order of precedence is as follows, Parenthesis -> Exponentiation -> Division -> Multiplication -> Addition -> Subtraction(PEDMAS). Division and Multiplication have the same order of precedence so as Addition and Subtraction.
13. Which of the functions do we use to import the Python random module?
(a) import random
(b) import random.h
(c) All of the above
(d) None of the above
Ans: (a)
Explanation: The random module in python is used to provide random numbers between specified ranges, we import it using the option (a).
14. Which among the following is NOT a Python string method?
(a) capitalize()
(b) find()
(c) isalnum()
(d) search()
Ans: (d)
Explanation: capitalize() converts the first string character into text. find() searched the string for a specified value and returns its position, isalnum() returns true if all characters are alphanumeric. Other string functions include casefold, endswith, format, isdigit, asascii, join among others.
15. Which of the following is a tuple method in Python?
(a) indexOf()
(b) count()
(c) index()
(d) get()
Ans: (b), (c)
Explanation: The count function returns the number of occurrences of a value in a tuple. index() searched the tuple for a specified value and returns its position. These are the only two python tuple built-in functions.
16. Which of the functions allows us to find the python version we are working on?
(a) sys.version
(b) sys.version(1)
(c) sys.version(0)
(d) None of the above
Ans: (a)
Explanation: sys is a module in python that provides functions and variables used to manipulate different parts of the python runtime. To get the python version we use option (a).
17. Which among the following is NOT a Python sys module function?
(a) sys.argv
(b) sys.exit
(c) sys.exitfunc
(d) sys.stdin, sys.stdout, sys.stderr
Ans: None of the above
Explanation: sys.argv refers to a list containing a=command-line arguments. sys.exit safely exists the program in case of any exceptions, sys.exitfunc is used to clean up actions after the program exists. sys.stdin, sys.stdout, sys.stderr are used for input, output, and error messages respectively.
18. What type is returned by the trunc() function in Python?
(a) bool
(b) string
(c) int
(d) float
Ans: (c)
Explanation: The math.trunc() function returns the truncated integer part of a number. It does not round the number up or down but rather removes the decimals.
19. Who created the Python Programming Language?
(a) Satoshi Nakamoto
(b) Guido van Rossum
(c) Wick van Rossum
(d) None of the above
Ans: (d)
Explanation: The python programming language was designed by a Dutch programmer by the name of Guido van Rossum.
20. Which programming language paradigm does Python follow?
(a) Object Oriented
(b) Structured
(c) Functional
(d) All of the above
Ans: (d)
Explanation: Python is an interpreted language that supports object-oriented, structured, and functional programming paradigms.
21. Select a feature of the Python DocString.
(a) Can be accessed using the '__doc__' attribute.
(b) Can be used to associate documentation with modules, functions, classes, and methods.
(c) Required in all python functions
(d) All of the above
Ans: (d)
Explanation: Documentation strings in python are used to document python programs better. They make the code easy to read and understand.
2. Which among the following is NOT a Python keyword?
(a) lambda
(b) new
(c) nonlocal
(d) yield
Ans: (b)
Explanation: 'new' is NOT a keyword in Python. The 'lambda' keyword is used to define a function that does not have a name for example;
f = lambda a: a + 9
print(f(4))
The output of the above snippet is '13'. The function, in this case, is 'f'. The 'nonlocal' keyword in Python allows us to modify the global scope. The 'yield' keyword is used like a 'return' keyword. It specifies what is returned from a function.
23. What is the function of the built-in 'type' function?
(a) To determine the class of any value
(b) To determine the object name of any value
(c) To determine the class description of any value
(d) To determine the file name of any value.
Ans: (a)
Explanation: type is used to get the class name of any value. For example, to get the class name,me of a string we write;
>>> type("string")
24. Which are the two main types of functions in Python?
(a) internal and external
(b) built-in and custom
(c) user-defined and custom
(d) built-in and user-defined
Ans: (d)
Explanation: In Python we have two function types built-in functions are built into the Python programming languages, these include max() min() among others, user defined functions are functions created by the programmer.
25. Which among the following is NOT a type of inheritance in Python?
(a) Single-level
(b) Multiple
(c) Double-level
(d) Multi-level
Ans: (c)
Explanation: Double-level is NOT a type of inheritance in Python. Single inheritance enables a subclass or derived class to inherit properties from a parent class. In multiple inheritances, a child can inherit from more than a single parent class, in multi-level inheritance the transfer of properties or characteristics is done in more than one class hierarchically.
26. Which among the following is NOT a Python dictionary method?
(a) copy()
(b) get()
(c) key()
(d) values()
Ans: None of the above.
Explanation: copy() returns a copy of the dictionary, get() returns the value of the specified key, key() returns a list of all the dictionary's keys and value() returns a list of all values in the dictionary.
27. What is pickling in Python?
(a) Converting python byte stream into a python object hierarchy
(b) Converting a python object hierarchy into a byte stream
(c) Converting a list into a data table
(d) Converting a data table into a list
Ans: (b)
Explanation: We use pickling to serialize and deserialize Python object structures. In this case, pickling involves converting a python object hierarchy into a byte stream after which we store it in a database/file.
28. Which is NOT a python file method?
(a) read()
(b) flush()
(c) seek()
(d) write()
Ans: None of the above
Explanation: We use read() and write() to return the file contents and write to a file respectively. flush9) is used to flush the internal buffer and, seek() to change the file position.
29. Which is NOT a Python set Method?
(a) union()
(b) intersection()
(c) issubset()
(d) difference()
Ans: None of the above
Explanation: union() function return a set containing the union of sets, intersection keyword returns a set of intersections between two or more sets, issubset returns true if s set contains another set, and difference() returns a set containing the difference between two or more sets.
30. Which among the following is NOT a Python keyword?
(a) pass
(b) raise
(c) not
(d) async & await
Ans: None of the above.
Explanation: All of the above are Python keywords. The 'pass' keyword is used as a placeholder for future code that we may write. The 'raise' keyword is used to raise an exception. The 'not' keyword is used to get the opposite Boolean value. The 'async' keyword is used with def keyword to create an asynchronous function similar to how we'd do it in Javascript. The syntax is as follows;
async def<function_name>(<function_parameters>):
# code here
'await' can only be used in asynchronous functions to wait for a return value. The syntax is as follows;
result = await <async_function_call>
31. Which of the following can be converted into a JSON object in Python?
(a) dist, list tuple, string, int, float
(b) True, False, None, int, string, float
(c) int string, float
(d) dict, list, tuple
Ans: All of the above.
Explanation: Python uses JSON to store and exchange data. We can parse JSON data using 'json.loads()' and convert to JSON using 'json.dumps()' method.
32. Which of the following is NOT used with pip?
(a) install
(b) uninstall
(c) list
(d) delete
Ans: (d)
Explanation: We use pip as a Python package manager. We install packages using pip install, delete them using pip uninstall and list them using pip list.
33. Which is NOT used in Python error handling?
(a) try
(b) except
(c) else
(d) finally
Ans: None of the above
Explanation: The try block allows us to test a block of code for errors, except allows us to handle the error if any, else block allows us to execute code when there is no error, and finally allows us to execute code regardless of the result from the previous steps.
34. Which is NOT a built-in Math function in Python?
(a) min & max
(b) abs & sqrt
(c) pow & sq
(d) ceil & floor
Ans: (c)
Explanation: min & max are used to get the minimum and maximum values. The abs function returns the absolute positive value of a specified number. The sqrt function returns the square root of a number, pow(x, y) returns x to the power of y, that is x ^ y. Ceil rounds up a number to the nearest integer while the floor rounds down a number to the nearest integer.
35. Which is NOT a Python list function?
(a) append
(b) remove
(c) sort
(d) push
Ans: (d)
Explanation: Append adds an item to the end of a list. Remove removes a specified item in a list, it takes the item as an argument. Sort sorts a list alphanumerically. Push is NOT a python list function.
36. Which among the following is NOT a python collection data type?
(a) List
(b) Tuple
(c) Set
(d) Dictionary
Ans: None of the above
Explanation: A list is ordered and mutable, we can have duplicates in a list which is NOT the case for a set, a set is unordered and mutable, and unindexed. A tuple is ordered and immutable. It also allows duplicates. Dictionaries are ordered and mutable, they don't have duplicate keys.
37. Which is NOT a Python data type?
(a) str
(b) int, float
(c) bool
(d) boolean
Ans: (d)
Explanation: In Python boolean types are represented by the 'bool' keyword. Other Python data types include NonType, bytes, bytearray, memoryview, set, dict, tuple, list, range, and complex.
38. Which among the following is a special symbol used for passing arguments in Python?
(a) *args
(b) args
(c) *kwargs
(d) kwargs
Ans: (a), (c)
Explanation: *args non-keyword arguments and *kwargs keyword arguments are used as function arguments when we are not sure of the number of arguments a function takes.
39. Which among the following is NOT a Python keyword?
(a) is
(b) in
(c) as
(d) of
Ans: (d)
Explanation: 'of' is NOT a python keyword. Unlike the '==' which checks for equality of two objects the 'is' keyword is used to check the identity of an object. The 'in' keyword is used to check for membership while the 'as' keyword is used to alias an imported module in a Python file.
40. Which of the following is NOT used in Python file handling?
(a) "r"
(b) "a"
(c) "w"
(d) "x"
Ans: None of the above
Explanation: We use "r" to read a file, "a" to append to the file, "w" to write to the file, if a file is not available then a new one is created, "x" creates a specified file. It returns an error if the file already exists.
41. Which of the following is NOT a number in Python?
(a) int
(b) float
(c) complex
(d) uint
Ans: (d)
Explanation: Uint represents a number in Solidity programming language. Int represents integers, float represents floating point numbers and complex numbers are represented by "x + yi".
42. Which is NOT a category of Python operators?
(a) Arithmetic
(b) Assignment
(c) Bitwise
(d) Logical
Ans: None of the above
Explanation: Other operators in Python are identity operators, membership operators, and comparison operators.
43. Which among the following is NOT a Python built-in function?
(a) compile()
(b) encode()
(c) id()
(d) dir()
Ans: (b)
Explanation: The encode() function is a string method/function that returns an encoded version of a string. The compile function returns a specified source as an object that is ready to be executed. The dir() function returns a list of an object's properties and methods. The id() function returns the id of an object.
44. Which among the following is NOT a python list method?
(a) index()
(b) count()
(c) insert()
(d) pop()
Ans: None of the above
Explanation: index() returns the index of the first element with the specified value. count() returns the number of elements with the specified value, insert() adds an item with a specified value, and pop() removes an element from a specified position in the list.
45. Which among the following is used for single-line comments in Python?
(a) {}
(b) #
(c) //
(d) //
Ans: (b)
Explanation: In Python we use the '#' character to comment single lines. To comment out multiple lines we write as follows;
# single commented line
x = 10
"""
multiple commented
\ lines here
"""
y = 17
46. What is the maximum length of an identifier in Python
(a) 100 characters
(b) 20 bytes
(c) 100 bytes
(d) 10 bytes
Ans: None of the above
Explanation: Python identifiers are names we use to identify variables functions modules classes. In Python, identifiers don't have a maximum length.
47. Which among the following is used to create anonymous functions at runtime?
(a) def
(b) pypi
(c) lambda
(d) pip
Ans: (c)
Explanation: Lambda functions are anonymous in that they don't have a name. We create the using the 'lambda' construct. 'PyPI(Python Package Index)' is a repository for python software packages. We use 'pip' to install Python packages during development.
48. Which of the statements best describes a package in Python?
(a) A collection of python modules.
(b) A collection of files with python functions
(c) A set of programs using python modules
(d) None of the above.
Ans: (a)
Explanation: Packages in Python are a way of structuring python's module namespace.
49. Which among the following is NOT a Python built-in function?
(a) bin
(b) dict
(c) enumerate
(d) ping
Ans: (d)
Explanation: 'ping' is a Linux networking command that sends ICMP packets to discover if a host is Up/down. The 'bin' function in Python is used to print the binary version of a number. The 'dict' function returns a new dictionary. The enumerate function takes a collection as a parameter and returns it as an enumerated object.
50. Which is NOT a Python core data type?
(a) Class
(b) Lists
(c) Dictionaries
(d) Tuples
Ans: (a)
Explanation: Others include, integers, strings, floats, complex numbers, sets, and files.