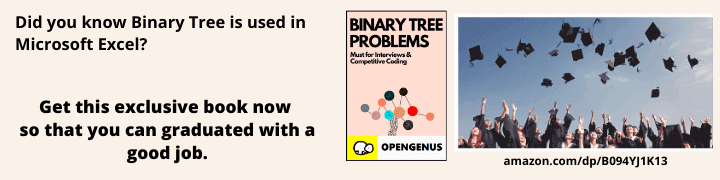
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored everything about functions in Python along with different types of functions that are available with Python code examples.
Table of contents:
- Introduction to Function
- Types of Functions in Python
- Anonymous Functions & Built-in Functions
- How to define User-Defined Function?
- Function Overloading
Introduction to Function
Functions basically means a piece/block of code that can be used again and again whenever the coder requires, it can be imported in any other programs that will only make that other program easy, less complex to run/understand, it actually provide a greater modularity for any code. They, are used to complete a specific task fluently.
We use function everywhere in our programming language, even the basic:
>>>print('hello world')
is using a built-in function of python to print the string hello world on the terminal.
Advantages of using Functions:-
- Less complex code, leading to easy understanding and fast debugging.
- Short code, as functions can imported in any other code.
- Saves time.
- The rest of the program is clean and narrow
Types of Functions in Python
The Different types of Functions in Python are:
- Built-in Functions
- User-defined Functions
- Anonymous Functions
1) Built-in Functions- Functions that come prebuild with the language like print(),help(),input().
2) User-defined Functions - Functions that are defined by the programmer according to his/her need.
3) Anonymous Functions- These functions are like any other user-defined, but the difference is the way we declare them, def() keyword is not used here, as they are nameless function. They are also called lambda funtions.
These functions can be defined using lambda keyword. These function are genrally used when a function is required for shorter amount of time. Generally used with map(), filter() functions.
Anonymous Functions & Built-in Functions
Syntax:-
lambda arguments:expression
Example:-
#to multiply two variables'''
multiply_numbers=lambda x,y:x*y
#main
print(multiply_numbers(4,5))
Output:-
20
Example with map() function:-
my_list = [1, 5, 4, 6, 8, 11, 3, 12]
new_list = list(map(lambda x: x ** 2 , my_list))
print(new_list)
Here, we are creating a new list where every element from original list has been raised to power 2.
How to define User-Defined Function?
User-defined functions in python start with the keyword def(), with all the parameters inside the parentheses
Syntax -
def function_name(parameter1 ,parameter2,......):
body
Example:
def multiply_number(x,y):
return(x*y)
here, we defined a function by the name multiply_number and pass two parameters x and y, and then by using return function we return their multiplication to the main function. it is never necessary for every function to have a return value, it all depends on the user programmer requirments.
Function without return type:-
def no_return_value():
print("Hello")
Here we defined a function no_return_value which has no parameter and also doesnt return any value, it just prints.If we call like then output will be:-
#main
no_return_value()
Output:-
Hello
Calling a user defined function:-
#main
x=int(input())
y=int(input())
calling=multiply_number(x,y)
print(calling)
here we are defining the main, intially we are taking tow input in two variables and passing these two varibales as argument to the defined function which when called will return their multiplication and then we are printing with the help of print function.
Flow of the program:-
def multiply_number(x,y): ###########1
return(x*y) ###########2
#main ###########3
x=int(input()) ###########4
y=int(input()) ###########5
calling=multiply_number(x,y) ###########6
print(calling) ###########7
All the lines have been given a number, lets understand its flow.
4-->5-->6-->1-->2-->7
first from line 4 & 5 we get the input from the user, then pass it on the function in line 6, then the function gets called therefore line 1 & 2 are exceuted after returning the value line 7 is excecuted and desired result is printed.
Function Overloading
Function are called methods if defines inside a class(). Thus we will address this as method overloading. Direct Function overloading in not supported by Python nor C++. Overloading in programming means a single function behave differently based on the parameters. Lets Understand with a simple example:-
class A():
def hell(self,x=none):
if x is not None:
print("Hell is"+x)
else:
print("Just Hell")
#main
obj=A()
obj.hell()
obj.hell('Bad')
Output:-
Just Hell
Hell is Bad
As, in the above example one can see same function is acting differently based on the parameters, this is simplest form of function overloading but not the most efficient.
One may also come across Operator Overloading, it simply means giving extended or updated meaning to existing operators, since we cant make new operators, again lets take a example to understand it better.
class Example():
def __init__(self,a,b):
self.a=a
self.b=b
def __mul__(self,different):
return self.a*different.a,self.b*different.b
#main
obj1=Example(5,4)
obj2=Example(2,6)
obj3=obj1*obj2
print(obj)
Output:-
(10,24)
Here, we defined a class Example with two method that are called automatically since (two underscore are used), here we have overloaded the mul function according to our need. In the main function we created two object of the defined class namely obj1 and obj2 and in the 3rd line we called the * operator where the magic happens i.e operation happens first argument of first object is multplied with first argument of second obj and so on. This __mul__
and many more like __add__
or __div__
are somtimes referred as MagicOperators.
With this article at OpenGenus, you must have the complete idea of Functions in Python.
Next Tasks:-
- Understand Classes and Object
- Understand self used in function declartion inside class
- Nested Function