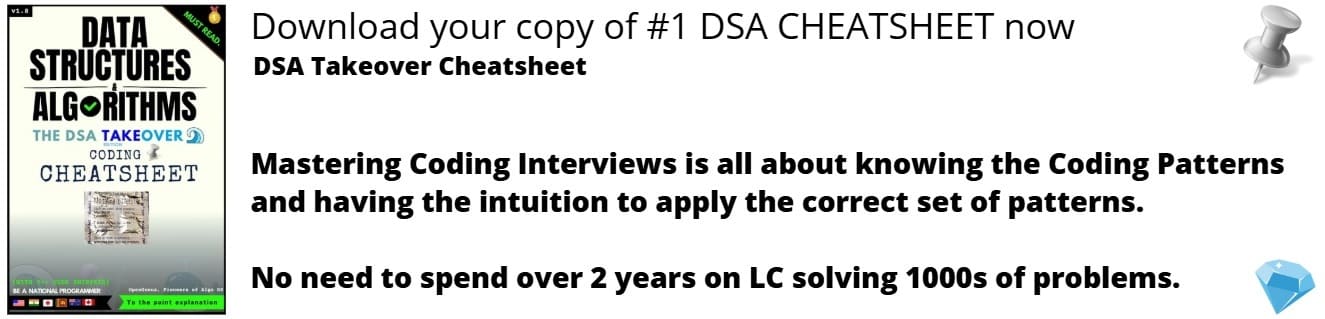
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
The browsers history is present in the history object. We can use the window part (window.history) or just simply history. It contains an array of URLs or links visited by the user.
Property of history object :
- length: It returns the length of the history URLs visited by user in that session.
⭐ Important : The length property returns atleast 1, beacuse the list includes the currently loaded page. This property can find out how many pages the user has visited in a browsing session. The array that comprises the browsers history starts from different position for different browsers like, Internet Explorer and Opera starts with 0 while Chrome, Firefox and Safari starts with 1. The maximum length of this property is 50 and is read-only.
Example of length property
<p id="p1"></p>
<script>
var result = window.history.length;
document.getElementById("p1").innerHTML =
"Currently, the value of <b>history.length</b> is <b>"+ result + "</b>";
</script>
Output:
Methods of history object :
- back(): It loads the previous page. It is as same as clicking back in the browser.
- forward(): It loads the next page. It is as same as clicking forward in the browser.
- go(): It loads the given page number in browser. history.go(distance) function is as same as pressing the back or forward button in your browser and specifying the page exactly which we want to load.
Example of history.back() method
<script>
function previousPage() {
window.history.back();
}
</script>
Output:
⭐ Important : This example will not work if the previous page does not exist in the history list.
Example of history.forward() method
<script>
function NextPage() {
window.history.forward()
}
</script>
Output:
⭐ Important : This example will not work if the next page does not exist in the history list. It works exactly same as forward button of your browser. If the next page doesn’t exist it will not work.
Example of history.go() method
go(4) has the same effect as pressing your forward button four times.A negative value will move you backwards through your history in a browser. go(-4) has the same effect as pressing your back button four times.
<script>
function NextPage() {
window.history.go(4);
}
</script>
Output:
⭐ Important : This example will not work if the next four pages do not exist in the history list.
Adding and modifying history entries
The history object comprises of history.pushState() and history.replaceState() methods, which allow us to add and modify history entries, respectively.
1. The pushState() method
pushState() method takes three parameters: a state object, a title, and (optionally) a URL. These parameters are described below:
- state object — The state object is associated with the new history entry created by pushState(). Whenever the user navigates to the new state, a popstate event is fired.
- title — Passing the empty string here should be safe against future changes to the method. Alternatively, we could pass a short title for the state to which you're moving. Mozilla Firefox currently ignores this parameter, although it may use it in the future.
- URL — The new history entry's URL is given by this parameter.
Example:
let stateObject = {
honey: "bee",
};
history.pushState(stateObject, "page 2", "bee.html");
Suppose "honey.html" executes the above JavaScript code. This will cause the URL bar to display "bee.html", but won't cause the browser to load bee.html or even check that bee.html exists. Suppose now that the user navigates to http://google.com
, then clicks the Back button. At this point, the URL bar will display bee.html and history.state
will contain the stateObj
. The popstate event won't be fired because the page has been reloaded. The page itself will look like bee.html.
2. The replaceState() method
history.replaceState()
is exactly like history.pushState()
except that replaceState() modifies the current history entry instead of creating a new one.
⭐ Note that this doesn't prevent the creation of a new entry in the browser history.
replaceState()
is particularly useful when we want to update the state object or URL of the current history entry in response to some user action.
Example:
history.replaceState(stateObj, "page 3", "bee2.html");
This will cause the URL bar to display bee2.html, but won't cause the browser to load bee2.html or even check that bee2.html exists. Suppose now that the user navigates to http://www.microsoft.com
, then clicks the Back button. At this point, the URL bar will display bee2.html. If the user now clicks Back again, the URL bar will display the first URL, and totally bypass bee.html.