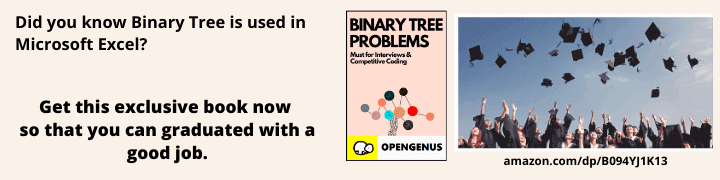
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Error encountered is: "TypeError: only integer scalar arrays can be converted to a scalar index".
This error comes when we handle Numpy array in the wrong way using Numpy utility functions. The fix is to refer the documentation of the Numpy function and use the function correctly.
Error:
TypeError: only integer scalar arrays can be converted to a scalar index
Table of Content:
- Solution
- Case 1: Error with np.concatenate
- Case 2: Error with np.ndindex
- See source of error in Numpy source code
Solution
The error you have faced is this:
TypeError: only integer scalar arrays can be converted to a scalar index
This means that the index you are using to refer to an array element is wrong. The index should always be an integer.
Despite this, due to flexibility of Python, you can use an array with one Interger element as an index. Internally, the array will be converted to a single Integer and will be used as an index.
This error is defined in Numpy library. So, you are using one of the functions in Numpy in a wrong way. You need to refer the Numpy documentation to confirm the API of the function which is giving an error.
You should be able to identify the issue. The most common issue is that a set of array needs to be passed as a list to a Numpy function. We will do not pass it as a list then, this error will be encountered.
# Not passing as list
array1, array2, ..., arrayN
# Passing as list
(array1, array2, ..., arrayN)
Case 1: Error with np.concatenate
Consider the following code snippet:
import numpy as np
a = np.zeros((1,2))
np.concatenate(a,a)
Using the above code snippet, we can reproduce the error. The error is as follows:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<__array_function__ internals>", line 6, in concatenate
TypeError: only integer scalar arrays can be converted to a scalar index
The solution is to use the array as a list:
np.concatenate((a,a))
Now, consider this Python code:
import numpy as np
a = [1,2]
np.concatenate(a,a)
This code looks simple and has an issue as well. The issue is different and is that a is a list. The error will be as follows:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "<__array_function__ internals>", line 6, in concatenate
TypeError: 'list' object cannot be interpreted as an integer
np.concatenate accepts Numpy arrays.
Case 2: Error with ndindex
We can get the same error with ndindex function of Numpy library.
Consider the following Python code:
import numpy as np
opengenus = np.ndindex(np.random.rand(128,128))
Error:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/local/lib64/python3.6/site-packages/numpy/lib/index_tricks.py", line 640, in __init__
strides=_nx.zeros_like(shape))
File "/usr/local/lib64/python3.6/site-packages/numpy/lib/stride_tricks.py", line 103, in as_strided
array = np.asarray(DummyArray(interface, base=x))
File "/usr/local/lib64/python3.6/site-packages/numpy/core/_asarray.py", line 85, in asarray
return array(a, dtype, copy=False, order=order)
TypeError: only integer scalar arrays can be converted to a scalar index
Note the error is same so the fix is same:
Fix:
np.ndindex(np.random.rand(128,128).shape)
Output:
<numpy.ndindex object at 0x7f1fe5f3cfd0>
See source of error in Numpy source code
This error comes from this function in Numpy source code (File: numpy/core/src/multiarray/number.c):
File:
numpy/core/src/multiarray/number.c
Code snippet from the Numpy code file:
static PyObject *
array_index(PyArrayObject *v)
{
if (!PyArray_ISINTEGER(v) || PyArray_NDIM(v) != 0) {
PyErr_SetString(PyExc_TypeError,
"only integer scalar arrays can be converted to a scalar index");
return NULL;
}
return PyArray_GETITEM(v, PyArray_DATA(v));
}
array_index is used whenever we refer to array index in Numpy. The function array_index is used as follows in the same file.
NPY_NO_EXPORT PyNumberMethods array_as_number = {
.nb_add = array_add,
.nb_subtract = array_subtract,
.nb_multiply = array_multiply,
.nb_remainder = array_remainder,
.nb_divmod = array_divmod,
.nb_power = (ternaryfunc)array_power,
.nb_negative = (unaryfunc)array_negative,
.nb_positive = (unaryfunc)array_positive,
.nb_absolute = (unaryfunc)array_absolute,
.nb_bool = (inquiry)_array_nonzero,
.nb_invert = (unaryfunc)array_invert,
.nb_lshift = array_left_shift,
.nb_rshift = array_right_shift,
.nb_and = array_bitwise_and,
.nb_xor = array_bitwise_xor,
.nb_or = array_bitwise_or,
.nb_int = (unaryfunc)array_int,
.nb_float = (unaryfunc)array_float,
.nb_index = (unaryfunc)array_index,
.nb_inplace_add = (binaryfunc)array_inplace_add,
.nb_inplace_subtract = (binaryfunc)array_inplace_subtract,
Conclusion:
This error comes when we handle Numpy array in the wrong way using Numpy utility functions. The fix is to refer the documentation of the Numpy function and use the function correctly.
With this article at OpenGenus, you must have the complete idea of solving this error. Happy debugging.