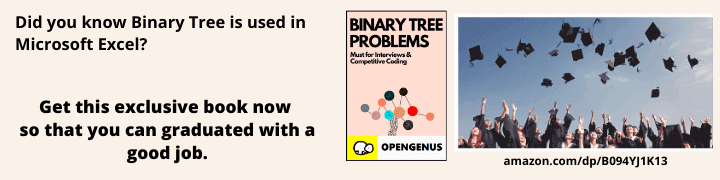
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, I have explained How I developed Search Bar at OpenGenus IQ using Google Programmable Search Engine. I have explained the challenges faced along with solution and code snippets.
Table of contents:
- Introduction
- General layout of the feature (The Modal)
- GCSE Search
- The openmodal() Function
- Home Page Search Feature
- Article Page Search Feature
- Challenges/ Functionalities to pay special attention to
Introduction
The Search Bar features for the OpenGenus IQ as well as the article pages were developed using HTML, CSS and JavaScript.
We use a pre-existing service known as the "Programmable Search Engine" facilitated by Google to implement the integral search box and mechanism. All the HTML, CSS and JS that we add is used for designing the search feature, its proper placing on the page and some custom behavior of it. We do not have to code the searching mechanism itself.
General layout of the feature (The Modal)
The general idea of the feature is to use a button or trigger which opens a new modal on the screen, right at the point where the web page is presently at. The modal contains the search box provided by Google. The Modal opens when the user presses the search button or bar.
<style>
.w3-modal {
padding-top: 100px;
position: fixed;
display: none;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(0,0,0,0.4);
}
.w3-modal-content {
margin: auto;
background-color: #fff;
position: relative;
width: 80%;
}
.w3-container{
padding: 0.01em 16px;
}
.w3-button {
position: absolute;
right: 0;
padding-right: 2.5%;
top: 0;
background: rgb(255, 255, 255);
padding-top: 2.5%;
}
@media screen and (min-width: 600px) {
.w3-modal-content {
width: 70%;
}
@media screen and (min-width: 800px) {
.w3-modal-content {
width: 60%;
}
}
</style>
<script async src="https://cse.google.com/cse.js?cx=ecbe1fb6c584cd3ff"></script>
<!-- please change the above script to your official opengenus ID -->
<div id="id01" class="w3-modal" style="z-index: 500; max-width: 0em 3em;" tabindex="-1" >
<div class="w3-modal-content">
<div class="w3-container">
<button onclick="document.getElementById('id01').style.display='none'"
class="w3-button">×</button>
<br><br>
<!-- <div class="gcse-search"></div> -->
<div class="gcse-searchbox"></div>
<div class="gcse-searchresults"></div>
</div>
</div>
</div>
<script>
function openmodal() {
document.getElementById('id01').style.display='block';
document.getElementById("gsc-i-id1").focus();
}
</script>
<style>
.gssb_c .gsc-completion-container{
position: fixed;
width: fit-content !important;
}
</style>
GCSE Search
In the above code snippet,
<script async src="https://cse.google.com/cse.js?cx=ecbe1fb6c584cd3ff"></script>
and
<div class="gcse-searchbox"></div>
<div class="gcse-searchresults"></div>
are the necessary code to implement the google custom search engine (gcse). Detailed documentation regarding the Google Custom Search Engine can be found here.
The modal looks like this:
When the user tries to type the search query in the search box, Google provides a default auto-complete option, which looks like this:
The CSS styling added after the modal in the code snippet
<style>
.gssb_c .gsc-completion-container{
position: fixed;
width: fit-content !important;
}
</style>
has been added to ensure that this auto-complete suggestions box always stays fixed on the page and its width is dynamic to accommodate the length of the suggestion. Without this style tag, the completion container would stay at the top of the page if you scroll down while searching OR it won't be visible at all if the search modal is called at a later point of the web page.
The openmodal() Function
The openmodal()
function defined in the script
<script>
function openmodal(){
document.getElementById('id01').style.display='block';
document.getElementById("gsc-i-id1").focus();
}
</script>
is called on by the search button/ input box when it is clicked. This function makes the modal visible and transfers the focus to the gcse input box, which has the id "gsc-i-id1". As we proceed, we will be adding this function in the onClick attribute of our search buttons.
Home Page Search Feature
For the home page, we use a search bar, which looks like this:
The code and the styling is as follows:
<style>
.searchbar {
width: 360px;
height: 36px;
left: 461px;
top: 399px;
margin-bottom: 1em;
}
.searchbartext {
width: 360px;
height: 36px;
left: 461px;
top: 399px;
border: none;
font-family: Noto Sans HK;
font-style: normal;
font-weight: normal;
font-size: 14px;
line-height: 20px;
padding-left: 5%;
color: #ffffff;
background: rgba(255, 255, 255, 0.4);
box-shadow: 0px 4px 4px rgba(0, 0, 0, 0.25);
border-radius: 100px;
}
input:active {
border: none;
}
.gsc-control-cse {
background-color: #F4F8FB;
border: 1px solid #F4F8FB;
}
::placeholder{
color: #ffffff;
}
.btn-default{
position: absolute;
width: 17.62px;
height: 16.85px;
margin-left: -2em;
margin-top: 0.1em;
background: transparent;
}
@media screen and (max-width: 417px){
.btn-default{
margin-left: -14%;
margin-right: 0%;
}
.searchbartext {
margin-left: 2.5%;
margin-right: 2.5%;
width: 80%;
}
</style>
<center>
<form class="searchbar" role="search" method="get">
<input
autocomplete="off"
name="q"
placeholder="Search..."
title="search here"
type="text"
id="name"
onclick="openmodal();"
class="searchbartext"
/>
<button type="submit" disabled class="btn btn-default">🔍</button>
</form>
</center>
Note the onClick attribute in the input box, which calls the Javascript function that we defined earlier. When the input box is clicked, the modal shows up, which can be then employed to search the required query.
We see that the search bar is put right in the center of the page. Since we're on the homepage, the search bar makes it accessible for users to search for the required topics/authors right from the homepage.
Article Page Search Feature
For the article pages, putting the search option was tricky, because leaving the search bar at the top of the page as you read the article diminished the usability of the search option. The entire purpose of the search option was to be accessible quickly to clear any doubts or reading related articles. So, for articles, we place the search bar in the navigation bar at the top of the page, beside the share buttons. Here's what it looks like:
The navigation bar is always present at the top of the page, so the user can use the search utility at any point of the reading.
We use the following code and styling to implement it:
<style>
.searchbar {
height: 36px;
margin-bottom: 1em;
margin-top: 1em;
margin-right: 1.5em;
}
.searchbartext {
height: 33px;
font-size: 17px;
padding-left: 4%;
mix-blend-mode: normal;
border: 2px solid rgb(163, 163, 163);
background-color: #ccc;
color: #ffffff;
box-sizing: border-box;
backdrop-filter: blur(4px);
border-radius: 10px;
margin-right: 1em;
}
#name11{
width: 10em;
}
@media screen and (max-width: 417px){
.searchbartext {
width: 80%;
}
#name11{
width: 80%;
}
}
</style>
<center>
<button id="name11" onclick="openmodal();" class="searchbartext"/>Search Here... 🔍</button>
</center>
<!--the following script has been added to change button text when size of window changes to make it responsive. -->
<script>
function buttonResponse(x) {
if (x.matches) {
document.getElementById("name11").innerHTML="Search";
}
else{
document.getElementById("name11").innerHTML="Search Here... 🔍";
}
}
var x = window.matchMedia("(max-width: 417px)")
buttonResponse(x)
x.addListener(buttonResponse)</script>
In the above code, we use some JavaScript to make the search button more responsive. Since, "Search Here... 🔍" is too long for smaller screens and overflows through the button boundaries, we replace the text with a simple "search" for smaller screens. This script is added after the search bar in the fucntion buttonResponse()
.
Challenges/ Functionalities to pay special attention to
- For the design aspect of the search bars, to get the suitable CSS styling for the page which went with the overall design of the page, I used Figma to design the bar. Figma was helpful in autogenerating the style apsects of the bars/button, such as the shadowing and the looks.
- To create the modal, I learned a lot from W3.CSS, a quality CSS framework developed by W3Schools.
- When working with GCSE, the simple basic divs
<div class="gcse-searchbox"></div> <div class="gcse-searchresults"></div>
that we write as changed into full fledged and intricately nested objects, full of tables, forms, buttons, etc. Inspecting exactly which div was influencing what aspect of the search feature was a significant challenge in getting the styling and functionality right, according to our needs. When adding the custom styling for the auto-completion container, it was tricky to find the exact class which it belonged to, because the auto-complete function would disappear the moment a different button was clicked. For this, I referred to this: this thread . Using a function on the console to freeze the DOM worked for me!
This was how the search feature @OpenGenus was developed!