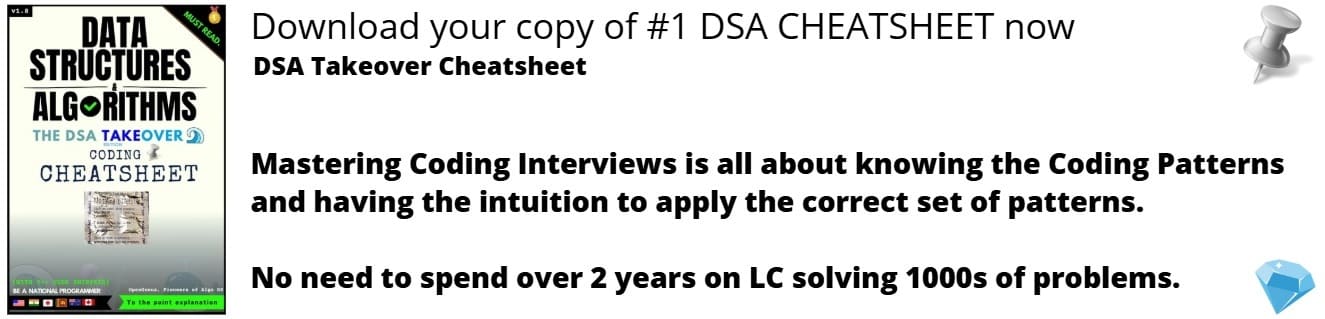
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the Jinja Template. Jinja is a modern, designer-friendly templating language for python, modeled after django templates and is widely used for execution.
In a template, the variables can be replaced by placing relevant values.
Example:
{{ fruit }} is the national fruit of {{ country }}
Fruit: mango and country: india
Mango is the national fruit of india
Template engines are more often used in web apps.
An HTML example:
<html>
<head>
<title>{{title}}</title>
</head
<body>
<h1>{{header}}</h1>
<p>{{body}}</p>
</body>
</html>
I hope this blog helps you understand basic functions used in django templating.
Table of Contents:
- Prerequisites and installations.
- Features and Delimiters
- Jinja conditional Statement Template
- Jinja Loop Template
- Jinja Assignments Template
- Jinja Template Inheritance
- Conclusion
1) Prerequisites and Installations
Jinja works with python 2.6.x, 2.7.x and >=3.3. If using python 3.2, old version of jinja needs to be used.
First install and setup jinja using virtual Environment:
pip install jinja2
and add it in requirements.txt file.
The simple jinja template code would look like:
>>> from jinja2 import Template
>>> t = Template("Hello {{ token }}!")
>>> t.render(token="Jinja")
u'Hello Jinja!'
2) Delimiters and Features
Jinja templates offer some basic programming functionalities such as variable substitutions, for loops, functions calls, filters, as well as the ability to extend base components.
Variable substitution: Variables can be substituted within templates by using a double curly brace around the variable name {{ variable }}
Tags: ForLoops and control flow (if/else)can be included within the template using tags. Tags come in the following syntax {% tag %}, the for loop is for instance implemented in the following way:{% for i in myList %} {{i}} {% endfor %}
Filters: filters can be used to perform some operations on variables, such as defining default value: {{ myVariable|default(âundefinedâ) }} ,
Lower case: {{ myVariable|lower }}
truncate: {{ myVariable | truncate(10)}}
join a list of string: {{ myList | join(â,â }}
Function calls: It is possible to call functions defined elsewhere in python call within Jinja templates.
There are different kinds of delimiters that are defined in jinja.
{% ... %} : for conditions, expressions that will be evaluated
{{ ... }} : for values to print to the template output
{# ... #} : for comments not included in the template output
# ... ## : for line statements
3) Jinja conditional statement template
First, we will look at how to write an âifâ condition.
{% if <condition> %}
condition is satisfied
{% else %}
condition is not satisfied
{% endif %}
There are several conditions we can use with âifâ. Example:
We create a template called truth.txt
{% if truth %}
This is true
{% else %}
This is false
{% endif %}
Then render it with the variable.
template = env.get_template(âtruth.txtâ)
output = template.render(truth=True)
print(output)
Output:
This is true
4) Jinja loop template
In web development, we can have cases when a list should be displayed on the page: registered users, for instance, or a simple list of options. In Jinja, we can use a for structure as bellow:
# Define data structure
my_list=[0,1,2,3,4,5] # a simple list with integer
In Jinja, we can iterate with ease, using a for block:
âŚ
<ul>
{% for n in my_list %}
<li>{{n}}</li>
{% endfor %}
</ul>
âŚ
5) Jinja Assignments template
We can use the set function to assign values.
Syntax: {% set variable = value %}
Example:
{% set count = 5%}
{% for value in list %}
{% set count = count + loop.index %}
{% endfor %}
{{count}}
When the above code has executed the value of the count will not get affected it will remain as 5.
6) Jinja template inheritance
Template inheritance allows you to create building blocks that you can combine. In this section we will be using a HTML as an example. We make a template called, header.html
<HEAD>
<TITLE>{{ title }}</TITLE>
</HEAD>
We make a template called, base.html Include the header.html
<HTML>
{% include 'header.html' %}
<BODY>
</BODY>
</HTML>
Now render:
template = env.get_template('base.html')
output = template.render(title='Page Title')
print(output)
Output:
<HTML>
<HEAD>
<TITLE>Page Title</TITLE>
</HEAD>
<BODY>
</BODY>
</HTML>
Now we will enable child templates to use the base.html with blocks.
<HTML>
{% include 'header.html' %}
<BODY>
{% block content %}{% endblock %}
</BODY>
</HTML>
We make a template called, child.html That extends the base.html template.
{% extends "base.html" %}
{% block content %}
<p>
{{ body }}
</p>
{% endblock %}
Now render this new template.
template = env.get_template('child.html')
output = template.render(title='Page Title', body='Stuff')
print(output)
Output:
<HTML>
<HEAD>
<TITLE>Page Title</TITLE>
</HEAD>
<BODY>
<p>
Stuff
</p>
</BODY>
5) Conclusion
As a summary, I have discussed some basic important functions and their usages in Jinja templating.
In this post we learned:
- Installations in jinja
- All its Features and Delimiters
- Jinja conditional statement, loops and assignment template
- Jinja template inheritance
Question
Go through this code:
{% for color in colors %}
{{ color }}
{% endfor %}
template = env.get_template('rainbow.txt')
colors = ['red', 'green', 'blue']
output = template.render(colors=colors)
print(output)
What will be the output of the above code?
With this article at OpenGenus, you must have a strong idea of a jinja template and will be able to implement the same according to your interest.