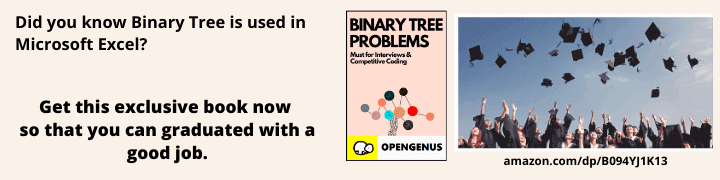
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In a simple way a Library is collection of builtin functions.One of the header file of standard C library is "math.h".As the name itself suggests,It defines various mathematical functions.The noticable thing is that all the arguements and return types of the functions of this header file is double.In this post we will discuss about power and exponentiation functions in this header file.
Table of contents:
- double pow(double x,double y)
- double exp(double x)
double pow(double x,double y)
Unlike Python and other programming languages,C doesn't have power operator.So we use a builtin function to compute such operations.pow() is a built in function in math.h header file,which takes two doubles as input and returns a double.The function prototype looks like thisdouble pow(double x,double y).Takes two doubles x and y i.e. base and exponent respectively.Here,x is raised to the power of y i.e. x^y.Let us consider an example to know how it works.
Input: 2.0 3.0
Output: 8.00
The function finds the value of 2.0 raised to the power of 3.0 (2.0^3.0) which is equal to 8.00 and returns the result.
Input: 5.0 4.0
Output: 125.00
The function finds the value of 5.0 raised to the power of 4.0 (5.0^4.0) which is equal to 625.00 and returns the result.
Lets write a code to implement the above example:
#include<stdio.h>
#include<math.h>
int main(){
double x,y;
printf("Enter the base and exponent values");
scanf("%lf %lf",&x,&y);
double result=pow(x,y);
printf("The Power value is %.2lf",result);
return 0;
}
output:
Enter the base and exponent values 3.0 4.0
The Power value is 81.00
As a learner,think about some interesting cases like,
1.does pow() work for negative inputs?
2.What if base or exponent is negative?
Now try passing negative values to the function and verify them.
The answer for the first question is YES.
pow() works for negative inputs as well.
To understand answer for the 2nd question,u need to have some mathematical knowledge.
Basically,when a exponent is negative i.e. (x^-y),it can be written as 1/(x^y).pow() function handles that case too.
Input: 2.0 -3.0
Output: 0.125000
The value of 2.0^-3.0 is equal to 1/8 which is equal to 0.125
If the base is a negative value,simply vwe get a positive value for even powers and negative for odd powers.i.e
Input: -2.0 3.0
Output: -8.000000
the situation can be explained as -2*-2*-2 which is equal to -8.
double exp(double x)
The function is used to find exponential of given value.exp() is also a built in function defined in "math.h" header file.It takes a parameter of type double and returns a double whose value is equal to e raised to the xth power i.e. e^x.Same as pow(),we have to include math.h header file in our program to access the function.Its function prototype looks like double exp(double x);.Let us consider an example to know how it works.
Input: 1
Output: 2.718282
The function finds the value of e raised to the power of 1.So we get the value of e which is equal to 2.718282.
Input: 5
Output: 2.718282
The value when e is raised to the power of 5 is e^5 ie 148.413159
The function exp() can also be written as pow(e,x),where the value of e is 2.718282.
Let's write a program to implement the abouve function.
#include <stdio.h>
#include <math.h>
int main()
{
double x;
printf("Enter the value of exponent");
scanf("%lf",&x);
double result=exp(x);
printf("\nThe Exponential value is %lf",result);
return 0;
}
Output:
Enter the value of exponent 5
The Exponential value is 148.413159
Similarly,if we pass negative parameters the function still works super fine.
When the input is negative,the function has to return e^-x,which can be written as 1/(e^-x).
Input: -5
Output 0.006738
We know that e^5 is 148.413159 as we done before,then the value of e^-5 will be 1/148.413159 which is equal to 0.006738.
Thanks for reading this article at OpenGenus :), Have a good day.