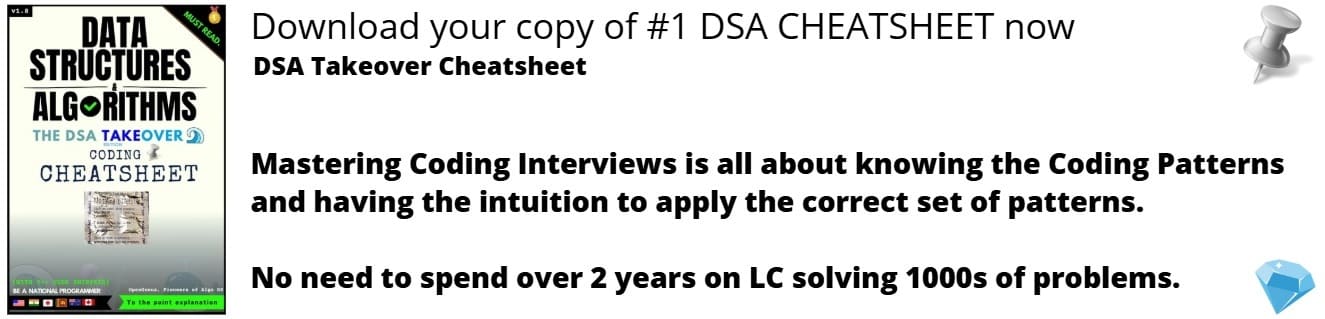
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
These are some of the most tough Interview questions on C Programming which tests your knowledge on how well you know the language. Even if you are not a master of C, you must try it once and get your personal score to check how good you are in C.
Bookmark this page 📌 so that you do not miss it at a later point when you should try it again.
The guide to evaluate your score:
Score | Level | Note |
---|---|---|
20 to 25 | Excelent | You have a strong hold in C concepts |
15 to 19 | Good | You are on the right path towards expertise |
10 to 14 | Nice | You have a strong base in C |
0 to 9 | Okay | On your way to master the basics |
Keep a note of how many questions you get right.
Which of the two is faster? 0==1 or 0==2
Both are equal
0==1
0==2
Runtime variation
One common misconception is that bitwise comparison takes place one bit at a time. In actual systems, all 8 bits of a byte are compared at a same time, so 1 or 2 makes no difference and both are same in terms of performance.
Inline keyword is used to define a function whose contents will be placed at the point, the function is called. It has another use. What is it?
Change linkage behaviour
Optimize code performance
Remove dependencies
Make code portable
This is important as C has two separate steps. In one step, the code is compiled and in the next step, the code is linked with relevant code snippets like libraries and common functions.
As in extern, function is not linked, it changes the linkage behaviour.
As in extern, function is not linked, it changes the linkage behaviour.
C and C++ are, often, considered to be similar. What is the major difference between the two programming languages?
C++ is OOP, C is not
C++ has garbage collection
C++ is faster
C++ has more optimizations
The major difference is that C++ is an Object Oriented Programming language (OOP) while C is a general-purpose imperative programming language. Due to this, it is difficult to design software systems based on object design in C.
There are alternatives in C like struct and union which makes the design of class like design possible to some extend.
There are alternatives in C like struct and union which makes the design of class like design possible to some extend.
char (*x) (char*);
In the above code snippet, what is "x"?
pointer to function
pointer to char pointer
pointer to char
character pointer
x is a pointer to a function that takes char* as a function parameter and returns char.
C is not OOP but struct and union allows one to design class like design. What is the major difference between struct and union features in C?
Union saves all its variables in same location
Union is more space efficient
Struct supports different data types
Struct supports pointer
The major difference is that in struct, all variables are stored in a block of memory that is identified by a name while in union, all variables are stored in the same memory location.
So, union has only one valid value at a time and is useful to represent a variable that can have different datatype at different points. On the other hand, struct is more close to the idea of a standard class.
So, union has only one valid value at a time and is useful to represent a variable that can have different datatype at different points. On the other hand, struct is more close to the idea of a standard class.
C did not have a boolean data type. In C99 (release in 1999), it was first supported. What is the new boolean data type named?
_ Bool
bool
boolean
Boolean
`_ Bool` is the original boolean data type. It was named as such to avoid breaking existing code as many developers developed work-arounds with their own version of boolean.
Hence, the bool data type in C requires us to import a header file.
Hence, the bool data type in C requires us to import a header file.
Learn to use the actual boolean data type in C
Boolean datatype only needs 0 or 1 value which can be represented by a single bit. What is the memory size of boolean data type in C?
1 Byte
1 bit
2 bit
16 Byte
Though Boolean data type needs only 1 bit, it has to use 1 byte (that is 8 bits) as in Computing systems, 8 bits or 1 byte is the smallest unit of memory that can be used. Operations can be performed on bits but in terms of memory allocation, the lowest level one can access in 1 byte.
So, in every boolean variable, 1 bit is used and the other 7 bits are vacant.
So, in every boolean variable, 1 bit is used and the other 7 bits are vacant.
C programming language has developed over the years and have many different standards like C11 is 2011. When was the first version of C released?
1972
1980
1999
2000
C Programming Language was a successor to B Programming Language and was developed by Ken Thompson and Dennis Ritchie in early 1970s and released in 1972.
The focus was to have more control on lower level system design.
The focus was to have more control on lower level system design.
What is the difference between #include"..." and include<...>?
#include"..." searches in current directory and #include \<...> searches in standard fixed directories
Both are same
#include \<...> searches in current directory and #include"..." searches in standard fixed directories
#include"..." is memory efficient
#include"..." searches in current directory and #include<...> searches in standard fixed directories. The include statement with quotes is used to import local libraries which may not be available in standard directories like /lib.
Memory leak is the case when the pointer to memory is destroyed but memory is not freed. Dangling pointer is a different memory issue. What is it?
pointer to freed memory
pointer that does not exist
a void pointer (void*)
Undefined pointer
Dangling pointer refers to the problem where a pointer is used which used to pint to a valid memory location but the memory has been deallocated in between. Due to this, the pointer does point to the same memory but it is not being used in the same way.
There can be another pointer variable that can point to the same memory location and hence, create conflicts in memory data.
There can be another pointer variable that can point to the same memory location and hence, create conflicts in memory data.
What is the issue with wild pointers?
Can point to non-existant memory
Can point to uninitialized memory
Can point to garbage value
Does not point to anything
As wild pointers are uninitialized pointers, it can point to any value and it can also, point to a memory location that does not exist. Due to this, program can crash if it is accessed. Hence, it is important to initialize pointers to NULL value.
If we pass a pointer variable to a function, the value can be changed. What are functions by default in C?
Pass by value
Pass by reference
Depends on variable
Depends on function scope
All functions in C are pass by value.
The reason why pointer variables can be changed is that the memory address is passed by value and following the address, the value can be changed. So, the memory address as pointed by the pointer does not change as defined by pass by value.
The reason why pointer variables can be changed is that the memory address is passed by value and following the address, the value can be changed. So, the memory address as pointed by the pointer does not change as defined by pass by value.
Extern keyword is used to declare a variable without defining it that is no memory is allocated. Where extern keyword is used?
In library files
In functions
In static functions
For global variables
Extern keyword is used to declare a variable without defining it that is no memory is allocated. It is used to declare variables in library files and in a client code where multiple libraries are imported, there should not be any conflict due to variables.
A variable can be declared multiple times but can be defined only once. Declaring means to specify the variable name and datatype. Defining means to allocate memory.
A variable can be declared multiple times but can be defined only once. Declaring means to specify the variable name and datatype. Defining means to allocate memory.
extern int a;
Learn everything about extern keyword in C
In International Obfuscated C Code Contest, there was an entry that had the following code snippet. What can you say about J?
#define o(X) r(copysign(1, X), exp2(J), exp2(J))
J must have been defined
J depends on value of copysign(1, X)
J is a C constant
exp2(J) is a constant value
J must have been defined before this statement. It can be a value or an expression that when computed results in a value.
In the same code snippet, what is meant by copysign?
Transfer sign of second value to first value
Transfer sign of first value to second value
Compares sign of the two values
Finds the difference between the two values
copysign is used to modify the sign of the first value based on the second value. If the second value is positive, the first value is made positive and if the second value is negative, the first value is made negative.
To run a system command from C code, what command can be used?
system()
setenv()
syst()
system.run()
system is a standard call in C that is used to run system commands directly from the C code. Note that the changes are reflected only in the child of current shell and is not observed as the program execution completes.
In the following code snippet, what is "x"?
custom_object& x = {1, "opengenus.org"};
reference to user-defined object
pointer to user-defined object
user-defined object
memory pointer
"&" is used after the data type to define references. References to variables are used to pass values in function without making a copy of the original data. This is efficient.
In the following compilation step, what will be the name of the output file?
gcc -std=c++14 -c -Iinc/ code.cpp -Llib/
code.o
a.out
code.so
code
The compiler option "-c" is used to get the object file from the given code file instead of directly generating the executable. Object files have an extension ".o".
In the Linux kernel, you will see the following code statement. What is meant by "-!!(e)" ?
#define BUILD_BUG_ON_ZERO(e) (sizeof(struct { int:-!!(e); }))
#define BUILD_BUG_ON_NULL(e) ((void *)sizeof(struct { int:-!!(e); }))
0 if e = 0 or else -1
0 if e = 0 or else 1
returns e
0 if e = 0 or else e
It is a way to check if expression e evaluates to 0 or not and raise a build error based on the value. In this case, -!!(e) will return 0 if e = 0 or else -1.
"assert" checks value at runtime. How to check values at compile time to avoid runtime failures?
Use -!!(e)
Use assert with extern
Use define macro
Not possible
Macros like -!!(e) are used to do compile-time checks and are an alternative to assert() which perform run-time checks.
In C Standard, the operator [] is defined as an arithmetic expression and used in array notation as well. What is the meaning of a[b]?
*(a+b)
a + *(b)
*a + b
a + b
a[b] is the b-th index in array a. So, the memory address of this element is denoted by *(a+b).
Hence, a[b] = b[a] where b is an integer.
Hence, a[b] = b[a] where b is an integer.
In the following code snippet, what is "x"?
int ** const x;
const pointer to pointer to int
pointer to const int
const pointer to const int
pointer
"x" is a const pointer to pointer to int. The expression can be seen as "int * (* const) x;"
What is the difference between calloc and malloc in C (for memory allocation)?
calloc initializes memory; malloc does not
malloc initializes memory; calloc does not
malloc for contiguous allocation
calloc for contiguous allocation
The only difference is that calloc initializes the memory it allocates to 0 and is slightly slower than malloc for this extra step. In case of malloc, memory may have garbage value.
size_t is an unsigned data type returned by the common function sizeof(). In which header file, size_t is defined?
stddef.h
stdlib.h
math.h
string.h
size_t is defined in stddef.h but it can be imported through stdlib.h as well.
Which of the following error code is returned by C code when "No such file or directory" error is encountered?
ENOENT
ENOFILE
EINVAL
ENOTFOUND
ENOENT stands for "Error NO ENtry" or "Error NO ENtity" and is used to denote the "No such file or directory" error.
What is your score? 🤔
Share with us by commenting down and let us know in which question you faced difficulty. Happy C mastering.