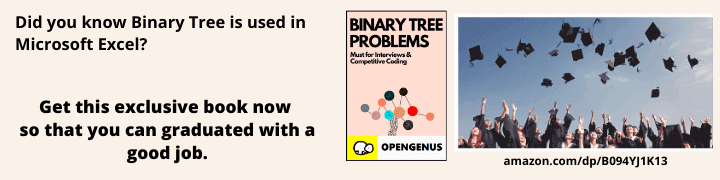
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have demonstrated how to design Tic Tac Toe Game as a webpage using HTML, CSS and JavaScript. We have presented all code and you should follow this mini-project.
Table Content
- Introduction
- How to function the game?
- Code Development
3.1. HTML file development
3.2. CSS file development
3.3. JavaScript file development and Implementation - Final Considerations
References
1. Introduction
The earliest reference to the Tic Tac Toe game dates from the excavations at the temple of Kurna (14th century BC) in Egypt.
Archaeological explorations indicated that this game developed in different parts of the world, and there are some versions (number of rows and columns varies).
Also, the game has different names depending on the region, for example, Tic Tac Toe (English-speaking countries) and Jogo da Velha (Brazil).
2. How to function the game?
Usually, two people can play the game at the same time. A player is represented by the symbol "X" and the other by a circle "O".
The game takes place on a board with four lines arranged horizontally and vertically (Figure 1).
Figure 1. Structure used in Tic Tac Toe.
The symbols "X" and "O" will be put between these lines.
The moves are alternate between player one and player two. The first to complete horizontally or diagonally with the symbol that represents herself/himself wins the game.
3. Code Development
The code uses HTML, CSS, and JavaScript. However, there are other ways to get an interactive Tic Tac Toe game.
The code was based on the work of Milne and CBFCursos.
3.1. HTML file development
Two containers were created (Figure 2):
<div id ="menu">, containing header information and a button to start/restart the game;
<div id="game">, id and class are used for styling the elements within the CSS file. In addition, id and onclick command, are need within the JavaScript file to make the game interactive.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tic Tac Toe</title>
<link rel="stylesheet" href="./assets/css/style.css">
</head>
<body>
<div id="menu">
<div id="whoStart">Who start: </div>
<button onclick="start()">Play</button>
</div>
<div id="game">
<div id="position1" class="gamePosition" onclick="play(1)"></div>
<div id="position2" class="gamePosition" onclick="play(2)"></div>
<div id="position3" class="gamePosition" onclick="play(3)"></div>
<div id="position4" class="gamePosition" onclick="play(4)"></div>
<div id="position5" class="gamePosition" onclick="play(5)"></div>
<div id="position6" class="gamePosition" onclick="play(6)"></div>
<div id="position7" class="gamePosition" onclick="play(7)"></div>
<div id="position8" class="gamePosition" onclick="play(8)"></div>
<div id="position9" class="gamePosition" onclick="play(9)"></div>
</div>
<script src="./assets/script.js"></script>
</body>
</html>
Figure 2. HTML file for the Tic Tac Toe interactive game.
3.2. CSS file development
The game frame uses id and class, previously informed in the HTML file (Figure 2).
In the CSS file, the background colors, space size and the "X" and "O" symbols were stipulated.
In the first part of the code (Figure 3), the game area was styled (colors, position, and size).
#game {
padding-top: 20px;
margin-left: 600px;
width: 400px;
height: 400px;
display: flex;
flex-wrap: wrap;
align-content: flex-start;
color: #FFF;
}
#menu {
text-align: center;
}
#whoStart{
margin-bottom: 10px;
}
.gamePosition {
width: 100px;
height: 100px;
margin: 0px;
padding: 0px;
cursor: pointer;
display: flex;
justify-content: center;
align-items: center;
font-size: 40px;
background-color: #CCC;
}
Figure 3. Stylization of the Tic Tac Toe area.
In the second part, the nine positions of the game board were stylized (format and colors) (Figure 4).
#position1 {
border-right: 1px solid #FFF;
border-bottom: 1px solid #FFF;
background-color: rgb(18, 52, 87);
}
#position2 {
border-right: 1px solid #FFF;
border-left: 1px solid #FFF;
border-bottom: 1px solid #FFF;
background-color: rgb(6, 8, 10);
}
#position3 {
border-left: 1px solid #FFF;
border-bottom: 1px solid #FFF;
background-color: rgb(18, 52, 87);
}
#position4 {
border-right: 1px solid #FFF;
border-top: 1px solid #FFF;
border-bottom: 1px solid #FFF;
background-color: rgb(6, 8, 10);
}
#position5 {
border: 1px solid #FFF;
background-color: rgb(18, 52, 87);
}
#position6 {
border-left: 1px solid #FFF;
border-top: 1px solid #FFF;
border-bottom: 1px solid #FFF;
background-color: rgb(6, 8, 10);
}
#position7 {
border-right: 1px solid #FFF;
border-top: 1px solid #FFF;
background-color: rgb(18, 52, 87);
}
#position8 {
border-right: 1px solid #FFF;
border-left: 1px solid #FFF;
border-top: 1px solid #FFF;
background-color: rgb(6, 8, 10);
}
#position9 {
border-left: 1px solid #FFF;
border-top: 1px solid #FFF;
background-color: rgb(18, 52, 87);
}
Figure 4.The stylization of the nine positions of the game board.
3.3. JavaScript file development and Implementation
To make the game interactive between the user (player) and the computer functions were developed. In the code, the computer plays with the user without analyzing the moves that the user does. The code randomly raffles a position. If it is empty, filled with a symbol, otherwise a new one is raffled.
Initially, creating the variables (Figure 5):
- game, saves played, and empty positions;
- table, control of visual elements;
- player, indicates the player's turn (0=player and 1=computer);
- playing, indicates the game state (true=loading and false=not loading);
- level, game difficulty level;
- moveComputer, indicates that it is the computer's move;
- starter, indicates who starts the game.
var game=[];
var table=[];
var player=0;
var verify;
var playing=true;
var level=1;
var moveComputer=1;
var starter=1;
Figure 5. JavaScript Code Variables.
Next step, the development of the function:
- function computerPlay (Figure 6):
a) responsible for making the computer play;
b) random move (random function). Does not analyze the opponent's move;
c) verifies if the game is running and implements the procedure checking the availability of the position within the board (filling with "X" or "O").
...
function computerPlay() {
if(playing) {
var row, column;
if(level==1){
do {
row=Math.round(Math.random()*2);
column=Math.round(Math.random()*2);
}while(game[row][column]!="");
game[row][column]="O";
}
verify=verifyVictory();
if(verify!=""){
alert(verify+" is the WINNER");
playing=false;
}
updateTable();
player=0;
}
}
...
Figure 6. Function computerPlay from JavaScript code.
- function verifyVictory (Figure 7):
a) implements a routine checking column, line, and diagonal, to detect if somebody wins. In case someone has won, it returns with the command "alert" if it was the "X" or the "O" who won the game and after the play finalizes.
...
function verifyVictory() {
var row, column;
//ROW
for(row=0; row<3; row++) {
if((game[row][0]==game[row][1])&&(game[row][1]==game[row][2])){
return game[row][0];
}
}
//COLUMN
for(column=0; column<3; column++) {
if((game[0][column]==game[1][column])&&(game[1][column]==game[2][column])){
return game[0][column];
}
}
//DIAGONAL
if((game[0][0]==game[1][1])&&(game[1][1]==game[2][2])){
return game[0][0];
}
if((game[0][2]==game[1][1])&&(game[1][1]==game[2][0])){
return game[0][2];
}
return "";
}
...
Figure 7. Function computerPlay from JavaScript code.
- function play (Figure 8):
a) verifies who is playing (player or computer);
b) checks if the game is stopped or not;
c) switch's structure, to receive the position played and test for each position the viability of being filled with the symbol "X";
d) verify if there is a winner.
...
function play(p) {
if((playing)&&(player==0)){
switch(p){
case 1:
if(game[0][0]=="") {
game[0][0]="X";
player=1;
}
break;
case 2:
if(game[0][1]=="") {
game[0][1]="X";
player=1;
}
break;
case 3:
if(game[0][2]=="") {
game[0][2]="X";
player=1;
}
break;
case 4:
if(game[1][0]=="") {
game[1][0]="X";
player=1;
}
break;
case 5:
if(game[1][1]=="") {
game[1][1]="X";
player=1;
}
break;
case 6:
if(game[1][2]=="") {
game[1][2]="X";
player=1;
}
break;
case 7:
if(game[2][0]=="") {
game[2][0]="X";
player=1;
}
break;
case 8:
if(game[2][1]=="") {
game[2][1]="X";
player=1;
}
break;
default:
if(game[2][2]=="") {
game[2][2]="X";
player=1;
}
break;
}
if(player==1){
updateTable();
verify=verifyVictory();
if(verify!=""){
alert(verify+" is the WINNER");
playing=false;
}
computerPlay();
}
}
}
...
Figure 8. Function play from JavaScript code.
- function updateTable (Figure 9):
a) function that does not contain any parameters. After a move (from player or computer), updates what appears on the computer screen.
b) runs through the game matrix and updates the board matrix according to the game matrix covered.
...
function updateTable(){
for(var row=0; row<3; row++){
for(var column=0; column<3; column++){
if(game[row][column]=="X"){
table[row][column].innerHTML="X";
table[row][column].style.cursor="default";
} else if(game[row][column]=="O") {
table[row][column].innerHTML="O";
table[row][column].style.cursor="default";
} else {
table[row][column].innerHTML="";
table[row][column].style.cursor="pointer";
}
}
}
}
...
Figure 9. Function updateTable from JavaScript code.
- function start (Figure 10):
a) responsible for initializing the matrix/template and the game;
b) indicates that the game has started;
c) indicates the first move;
d) contains the visual association with the variable board.
...
function start() {
playing=true;
moveComputer=1;
game=[
["","",""],
["","",""],
["","",""]
];
table=[ [document.getElementById("position1"),document.getElementById("position2"), document.getElementById("position3")],
[document.getElementById("position4"),document.getElementById("position5"), document.getElementById("position6")],
[document.getElementById("position7"),document.getElementById("position8"), document.getElementById("position9")]
];
updateTable();
if(starter==1){
starter=0;
player=starter;
document.getElementById("whoStart").innerHTML="Who starts: Player";
} else {
starter=1;
player=starter;
document.getElementById("whoStart").innerHTML="Who starts: Computer"
computerPlay();
}
}
window.addEventListener("load", start);
Figure 10. Function start from JavaScript code.
The result of the tests (Figure 11):
Figure 11. Illustrative code results.
4. Final Considerations
The code allows the user to play with the computer. But without the computer attacking or defending against the user's moves.
From this code, it is possible to implement a second level, in which the computer can better study the opponent's moves and defend itself. In this case, it would be necessary to implement a block (second level) in which the computer would perform the analysis of empty positions and fill them with the symbol "O" and then each position filled with "X" to be able to play in a more "realistic" way.
With this article at OpenGenus, you must have the complete idea of Design Tic Tac Toe Game (in JavaScript).