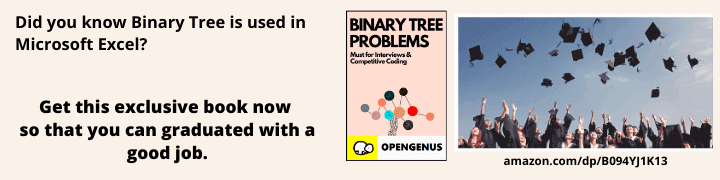
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
The Clipboard API provides a way to hook into the common clipboard operations of cutting, copying and pasting so that web application can adjust the clipboard data as required. There are two ways we can interact with the system clipboard:
The Document.execCommand() method and the modern asynchronous Clipboard API.
Interact with the clipboard
Mainly, there are two ways to interact with the clipboard. We can use the document.execCommand()
to trigger the "cut" or "copy" actions. The other option is to use the Clipboard API's Clipboard.writeText()
or Clipboard.write()
method to replace the clipboard's contents with a specific data.
1. execCommand() method
The document.execCommand() method comprises of "cut" and "copy" commands can be used to replace the clipboard's current contents with the selected material.
Important :
These commands can be used without any special permission if we are using them in a short-lived event handler for a user action (for example, a click handler).
Example:
<input id="input" type="text"/>
<button id="copy">Copy to Clipboard</button>
<script>
function copy() {
var copyText = document.querySelector("#input");
copyText.select();
document.execCommand("copy");
alert(copyText.value + " copied to clipboard.");
}
document.querySelector("#copy").addEventListener("click", copy);
</script>
Output:
For reading data from clipboard, we will need to have the "clipboardRead" permission established. This is the case even if we're using the "paste" command from within a user-generated event handler such as click or keypress.
Example:
<input id="output" type="text">
<button id="paste">Paste from clipboard</button>
<script>
function paste() {
var pasteText = document.querySelector("#output");
pasteText.focus();
document.execCommand("paste");
alert(pasteText.value);
}
document.querySelector("#paste").addEventListener("click", paste);
</script>
Output
2. Clipboard API
The Clipboard API adds more flexibility, in that we aren't limited to simply copying the current selection into the clipboard, but we can directly specify, what information to place into the clipboard.
For page scripts, the Permissions API's "clipboard-write"
permission is required. We can check for that permission using navigator.permissions.query()
.
Example:
<div>
<input type="text" class="to-copy">
<button class="write-btn">Copy to clipboard</button>
</div>
<script>
const writeBtn = document.querySelector('.write-btn');
const inputValue = document.querySelector('.to-copy');
writeBtn.addEventListener('click', () => {
if (inputValue) {
navigator.clipboard.writeText(inputValue)
.then(() => {
const lrt = inputValue.value;
inputValue.value = '';
if (writeBtn.innerText !== 'Copied!') {
const originalText = writeBtn.innerText;
alert(lrt+" Copied");
}
})
.catch(err => {
alert("Something went wrong");
})
}
});
</script>
Output:
The Clipboard API's navigator.clipboard.readText()
and navigator.clipboard.read()
methods let us to read arbitrary text or binary data from the clipboard. If we have the "clipboard-read" permission from the Permissions API, we can read from the clipboard easily.
Example:
<div>
<h6 class="clipboard-results">{{After pasting, text will appear here}}</h6>
<button class="read-btn">Paste from clipboard</button>
</div>
<script>
const readBtn = document.querySelector('.read-btn');
const resultsEl = document.querySelector('.clipboard-results');
readBtn.addEventListener('click', () => {
navigator.clipboard.readText()
.then(text => {
resultsEl.innerText = text;
})
.catch(err => {
alert("Something went wrong.");
})
});
</script>
Output:
{{After pasting, text will appear here}}
Copying and Pasting images from clipboard
The manipulation of the clipboard on the web is not an easy task, not even for plain text and much less for images. The content of the clipboard can't be easily retrieved using a method like clipboard.getContent. If we want to retrieve the images on the most updated browsers, we will need to rely on the paste event of the window.
The event can only be triggered by the user action on the document, namely pressing CTRL+ V.
When we press CTRL+ V and the current window is focused, this event is triggered. The thePasteEvent object that contains the clipboardData object is important. If the clipboardData object exists, then it will contain the items property (by default undefined if the clipboard is empty).
Example:
<p>
Focus this tab and press <kbd>CTRL</kbd> + <kbd>V</kbd>. The image on your clipboard will be rendered on the canvas !
</p>
<canvas style="border:1px solid grey;" id="mycanvas">
<script>
function retrieveImageFromClipboardAsBlob(pasteEvent, callback){
if(pasteEvent.clipboardData == false){
if(typeof(callback) == "function"){
callback(undefined);
}
};
var items = pasteEvent.clipboardData.items;
if(items == undefined){
if(typeof(callback) == "function"){
callback(undefined);
}
};
for (var i = 0; i < items.length; i++) {
// Skip content if not image
if (items[i].type.indexOf("image") == -1) continue;
// Retrieve image on clipboard as blob
var blob = items[i].getAsFile();
if(typeof(callback) == "function"){
callback(blob);
}
}
}
window.addEventListener("paste", function(e){
// Handle the event
retrieveImageFromClipboardAsBlob(e, function(imageBlob){
// If there's an image, display it in the canvas
if(imageBlob){
var canvas = document.getElementById("mycanvas");
var ctx = canvas.getContext('2d');
// Create an image to render the blob on the canvas
var img = new Image();
// Once the image loads, render the img on the canvas
img.onload = function(){
// Update dimensions of the canvas with the dimensions of the image
canvas.width = this.width;
canvas.height = this.height;
// Draw the image
ctx.drawImage(img, 0, 0);
};
// Crossbrowser support for URL
var URLObj = window.URL || window.webkitURL;
// Creates a DOMString containing a URL representing the object given in the parameter
// namely the original Blob
img.src = URLObj.createObjectURL(imageBlob);
}
});
}, false);
</script>
Output:
Focus this tab and press CTRL + V. The image on your clipboard will be rendered on the canvas !