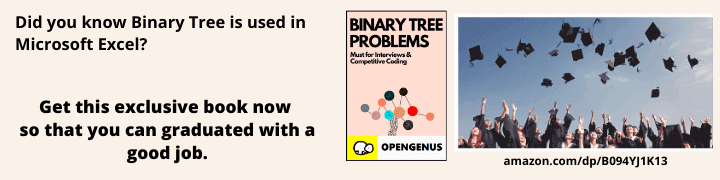
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to calculate the memory size of a struct in C and C++ Programming Language. To find the actual size, you need to understand two concepts of padding and packing. The size is not the sum of individual elements so read on.
Table of contents:
- Structure Padding
- Structure Packing
Let us explore the ideas with code examples and explanation.
Structure Padding
Computer stores structure using the concept of word boundary.
The size of a word boundary is machine dependant. In a computer with two bytes word boundary, the members of a structure are strored left_aligned on the word boundary.
Processor doesn't read 1byte at a time from memory.It reads 1 word at a time.
In 32 bit processor, it can access 4 bytes at a time which means word size is 4 bytes.
Similarly in a 64 bit processor, it can access 8 bytes at a time which means word size is 8 bytes.
Structure padding is used to save number of CPU cycles.
What we know is that size of a struct is the sum of all the data members. Like for the following struct,
struct A{
int n1;
int* n2;
char c1;
char *c2;
};
Size of the struct should be sum of all the data member, which is: Size of int n1+ size of int* n2 +size of char c1+ size of char* c2
Now considering the 64-bit system,
Size of int is 4 Bytes
Size of character is 1 Byte
Size of any pointer type is 8 Bytes
(Pointer size doesn't depend on what kind of data type they are pointing too)
So the size of the struct should be: (4+8+1+8)=21 Bytes
Let's see what compiler is giving using the sizeof() operator.
#include <stdio.h>
struct ABC {
int n1;
int* n2;
char c1;
char* c2;
};
int main()
{
struct ABC a;
printf("Size of struct ABC: %lu\n", sizeof(struct ABC));
printf("Size of object a: %lu\n", sizeof(a));
return 0;
}
Output of the above program is :
Size of struct ABC: 32
Size of object a: 32
It seems like the compiler took maximum size out of the data type and assigned the same memory to all data types.
It aligns till the boundary of maximum memory allocated. Here we find that max memory allocated is 8 Bytes, thus all the data members acquire 8 Bytes and the total size is 32 Bytes.
Is it like the number of data members * max datatype size?
The answer is no. Check the following structure which has the same members but the ordering is different.
struct B{
int* b;
char c;
int a;
char *d;
};
Consider this C code example:
#include <stdio.h>
struct BAC {
int* n2;
char c1;
int n1;
char* c2;
};
int main()
{
struct BAC b;
printf("Size of struct BAC: %lu\n", sizeof(struct BAC));
printf("Size of object b: %lu\n", sizeof(b));
return 0;
}
Output of the above program is :
Size of struct BAC: 24
Size of object b: 24
Compiler keeps aligning greedily and that's why it aligned char c & int a in the same row. When it tried to align char* d, it could not as only 3 bytes were left. But instead of char*, if it was char only then it would have aligned in the same line.
When we declare structure variables, each one of them may contain slack bytes and values stored in such slack bytes are undefined. Due to this, even if the members of two variables are equal, thier structures do not necessarily compare equal.
C therefore, does not permit comparison of structures.
Structure Packing
We can avoid the wastage of memory by simply writing #pragma pack(1)
#pragma pack(1)
#pragma
#pragma
is a special purpose directive used to turn on or off certain features.
#include <stdio.h>
#pragma pack(1)
struct ABC {
char a;
int b;
char c;
};
int main()
{
printf("Size of struct ABC: %lu\n", sizeof(struct ABC));
return 0;
}
Output of the above program is :
Size of struct ABC: 6
As int will take 4bytes and two character variable will take 1 byte each.
Now let us look upon some questions related to this topic.
Question 1
Which directive is used for structure packing ?
struct BAC {
char c;
int n;
char b;
};
Question 2
What is the size of the above structure?
With this article at OpenGenus, you must have the complete idea of how to calculate the size of structure (struct) in C and C++.