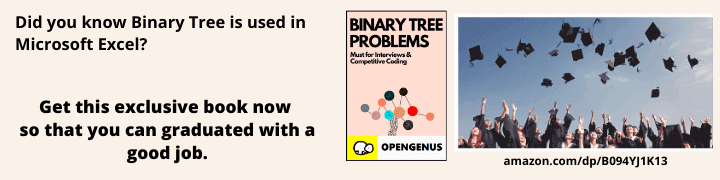
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 10 minutes | Coding time: 15 minutes
In this article you will learn how how to create PDF files out of your word document.Well up until recently, creating complex or elegant PDFs in Javascript has been challenging.Here I’m going to show you step-by-step the path of to create beautiful PDFs.
Before we dive further into the process why don't we learn what is word document or what is PDFs or what is the need to convert word document to PDFs.
Word Document is a popular word-processing program used primarily for creating documents such as letters, brochures, learning activities, tests, quizzes and students' homework assignments.DOC stands for DOCument file. A DOC file can contain formatted text, images, tables, graphs, charts, page formatting, and print settings.
The Portable Document Format (PDF)is a file format developed by Adobe in the 1990s to present documents, including text formatting and images, in a manner independent of application software, hardware, and operating systems
Yes, PDFs were created to avoid any changes that might occur due change in hardware or software which might have occurred to you if you had ever used Word document.Let's take a example,Whenever a make a word document and to a printing shop for the print the shop owner may have different OS,hardware or even software and when you get your printed document it is not what you saved at your computer maybe there is a large gap between line format is distorted or something else.So,that's where PDF come's to rescue.
Word to PDF approaches
There are three methods that I am going to discuss today which are very easy to use and produce excellent results. These are using:
- awesome-unoconv
- libreoffice-convert
- docx-pdf
1. awesome-unoconv
awesome-unoconv is nodeJS wrapper for converting Office files to PDF or HTML
REQUIREMENT
Unoconv is required, which requires LibreOffice (or OpenOffice)
You can install unoconv in linux operating system by using following command
sudo apt-get install unoconv
INSTALLATION
npm install awesome-unoconv
CODE
const path = require('path');
const unoconv = require('awesome-unoconv');
//Place your word file in source
const sourceFilePath = path.resolve('./word_file.docx');
const outputFilePath = path.resolve('./myDoc.pdf');
unoconv
.convert(sourceFilePath, outputFilePath)
.then(result => {
console.log(result); // return outputFilePath
})
.catch(err => {
console.log(err);
});
Now go to the terminal and run your command pdf will be created in current working directory with name "myDoc.pdf" (you choose any name you like).
This is one of the method to convert word document to pdf let's keep going.
2. libreoffice-convert
A simple and fast node.js module for converting office documents to different formats.
DEPENDENCY
Since,I am using linux please Install libreoffice in /Applications (Mac), with your favorite package manager (Linux), or with the msi (Windows).
INSTALLATION
npm install libreoffice-convert
CODE
const libre = require('libreoffice-convert');
const path = require('path');
const fs = require('fs');
const extend = '.pdf'
const FilePath = path.join(__dirname, './word_file.docx');
const outputPath = path.join(__dirname, `./example${extend}`);
// Read file
const enterPath = fs.readFileSync(FilePath);
// Convert it to pdf format with undefined filter (see Libreoffice doc about filter)
libre.convert(enterPath, extend, undefined, (err, done) => {
if (err) {
console.log(`Error converting file: ${err}`);
}
// Here in done you have pdf file which you can save or transfer in another stream
fs.writeFileSync(outputPath, done);
});
Since,This is only for libre-office you might not find it very usefull if you are using windows but for for linux/Mac operating system it is very popular.
Let's look at the last and third method to convert word document to PDFs
3. docx-pdf
It is a library that converts docx file to pdf and it is one of most optimal and quality wise best among the three and most easiest one also.
INSTALLATION
npm install docx-pdf
CODE
var docxConverter = require('docx-pdf');
docxConverter('./word_file.docx','./output.pdf',function(err,result){
if(err){
console.log(err);
}
console.log('result'+result);
});
Output should be output.pdf which will be produced on the output path your provided.
Question
Which one of the following is the correct command to install any module/package in your project?
If you want to see the project you can use this link to my github page.