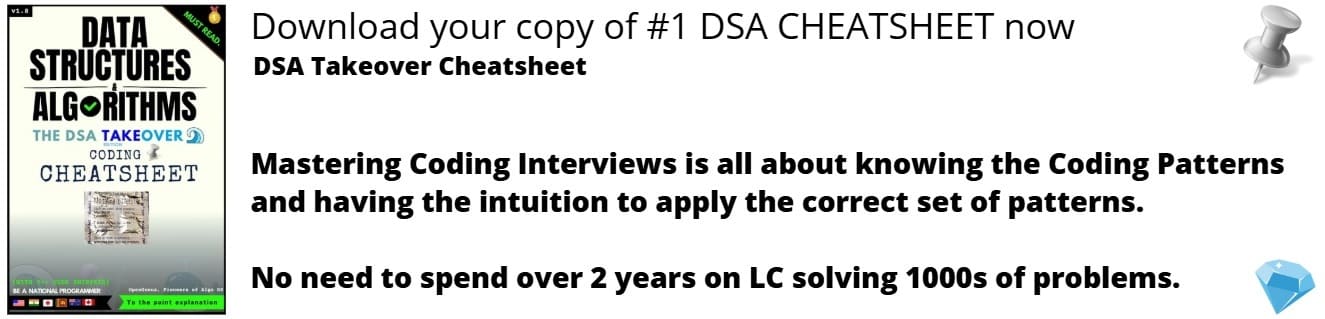
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
If you are a web developer or have developed a website earlier, you must have used javascript to make your website more functional and add more features. You also have thought about the long and repetitive syntax you have to type for adding simple CSS or selecting some HTML tag and changing some text in your project.
document.querySelector(“h1”).style.color = “red”.
You must have gone through this thought and have passed it, but not this guy, named “John Resig”. John thought that he needs to type and repeat so much of code again and again. So, to avoid this repetative code, he built a library in 2006 for javascript code to be written in short form and named it jQuery.
Today, jQuery is one of the most famous libraries and has been downloaded the most among other javascript libraries. jQuery has been used widely by nearly all web developers. It has helped to make the javascript code shorter and easier to read and type. It saves a lot of time and efforts.
In short, you can say, jQuery is javascript library that will prevent your fingers from breaking while coding.
E.g. to select the h1 tag using javascript, you type,
document.querySelector(“h1”)
With jQuery, it can be simply written as
jQuery.(“h1”)
Or, more simply, you can also write a shorthand way,
$(“h1”)
$(“h1”)
will work same as document.querySelector(“h1”)
. Here, the $ sign represents jQuery.
Setup for a project
There are different ways to incorporate jQuery in your projects and use the library. JQuery is available to use as an API, or you can download it as a local file.
The best way is to use its API with CDN (content delivery network). Scroll through the download JQuery page from the official documentation and click on Google CDN link from the “Using jQuery with a CDN” section.
You will be using google’s CDN to incorporate JQuery in your project as it is easier and faster to implement, and also, gives speedier loading.
When you open the Google's CDN link, you will find there are three links with different versions of jQuery. Just copy the latest version and add it at the end of your HTML page before the closing body tag.
Hurray! You are done incorporating jquery in your project.
Now, you can start working with jQuery in your project. Write all the jQuery code in the same javaScript file (.js), where you write all your javaScript code. It will work in the same way.
We will learn some basics of how to use jQuery in your project.
Note: You must have familiarity working with javaScript code in order to understand jQuery. Please make sure you have worked earlier using javaScript.
1. Selecting elements using jQuery.
To access a selector, use the jQuery symbol $, followed by parentheses ().
Basic syntax will look like this:
$("selector")
Below are some of the most commonly used selectors.
-
$(“h1”)
-
$(“p”)
-
$(“h1.title”)
-
$(“button”)
-
$(“.class”)
-
$(“#id”)
2. Applying methods to jQuery
You can access the DOM with jQuery using CSS syntax and implement action with a jQuery method.
A basic jQuery example follows this format.
$("selector").method();
You can manipulate CSS using selectors using .css() method.
$(“h1”).css(“color” , ”green”)
$(“h1”).css(“font-size” , ”20px”)
You can add and remove particular class to a element using addClass() and removeClass()
$(“h1”).addClass(“green-class”)
$(“h1”).removeClass(“green-class”)
You can change the value of an attribute of an element using the attr() method.
Basic syntax follows this format
$(“selector”).attr(“nameOfAttribute”,”newValue”)
$(“img”).attr(“src” , ”https://iq.opengenus.org”)
These are some the most commonly used methods.
3. jQuery events
To manipulate different elements on an HTML page, you can call a function inside an event that will trigger a method defined inside function body.
$(“selector”).event( function(){
Your method here
})
For E.g
$("h1").on("click", function(){
$("h1").css("color","purple")
})
4. jQuery Effects
jQuery effects allow you to add animations and manipulate elements on the page.
For E.g. To animate H1 tag with slide up when clicked on a button, then, we can use,
$("button").on("click", function(){$("h1").slideUp()
})
You can also use multiple effects on the same element.
$("button").on("click", function(){$("h1").slideUp().slideDown()
})
Below are some of the most commonly used effect methods.
- toggle() - Binds two or more handlers to the matched elements, to be executed on alternate clicks. show() and hide() are the relative one-way effects.
- fadeToggle() - Display or hide the matched elements by animating their opacity. fadeIn() and fadeOut() are the related one-way effects.
- slideToggle() - Display or hide the matched elements with a sliding motion. slideDown() and slideUp() are the related one-way effects.
- animate() - Perform a custom animation of a set of CSS properties.
You can find more effects on the official documentation page.
Conclusion
In this article, we learned what jQuery is and why you should use jQuery for web development. We learned how to setup and use jQuery in your projects the easier way. You must have got an idea how jQuery works and have basic understanding of using jQuery. From here, you can continue you learning process for more deeper understanding and follow up the official documentation to learn more.