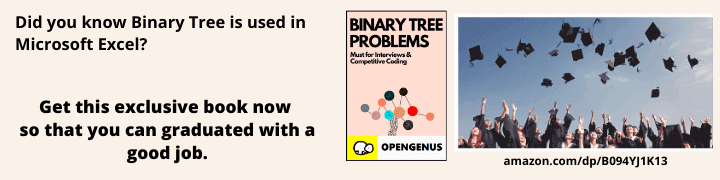
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents:
- Introduction
- Overall Design and Implementation Strategy
- Step 1: Setting up the HTML Structure
- Styling the Game
- Understanding the Javascript Code
- Final Product
- Conclusion
Introduction:
Hangman is a classic word-guessing game that challenges players to guess a hidden word by suggesting letters one at a time. We will be implementing the simple version of the game. A random word will be given to the player for guessing. And the player can guess a letter at each turn. If the letter is in the word, we show the matching places of the letter in the word. Else if the letter is not in the word, we draw the hangman body parts one at a time and display the wrong word so the user would not enter the wrong letter again. The game ends whenever the user correctly guesses the word and wins or when the hangman drawing is completed and the user loses.
In this article, we will provide a detailed guide on how to create a Hangman game using the provided HTML, CSS, and JavaScript code.
Overall Design and Implementation Strategy
We will be writing the HTML CSS and JS for this simple web application. Before diving into the specifics, we will look at the overall picture and discuss our implementation strategy.
We will use the HTML structure to display the hangman by using HTML lines. We will also put down the messages for notifying the users when game is done.
In the CSS code, we will decorate our HTML structure. We will style everything for the HTML structure. We will also define important style for hiding the notification for different stages of the game.
Finally in the JS code, we will implement the game logic. We will first randomly choose a word in the word list, then we will have the user try to enter a letter each time and check if it is in the word and do appropriate actions for if the letter is in the word or not. We will also check if the game ends each time the user finishes the entering letter action. Once we detect the game ends. The notficiation pops up and asks the user whether or not if they want to play again.
Now, we will get into the details of how to implement the game with code examples.
Step 1: Setting up the HTML Structure
Start by copying the provided HTML code into a new HTML file. The code defines the necessary elements for the game, such as the game container, word display, wrong letters container, popup, and notification. Make sure to save the file with an appropriate name. We will use Js in the later part of the tutorial to manipulate the DOM structure.
In the html we define the basic structure of the game. We also draw the stickman using the HTML lines.
<h1>Hangman</h1>
<p>Find the hidden word - Press a letter</p>
<div class="game-container">
<svg height="250" width="200" class="figure-container">
<!-- rod -->
<line x1="60" y1="20" x2="140" y2="20" />
<line x1="140" y1="20" x2="140" y2="50" />
<line x1="60" y1="20" x2="60" y2="230" />
<line x1="20" y1="230" x2="100" y2="230" />
<!-- head -->
<circle cx="140" cy="70" r="20" class="figure-part" />
<!-- body -->
<line x1="140" y1="90" x2="140" y2="150" class="figure-part" />
<!-- arms -->
<line x1="140" y1="120" x2="120" y2="100" class="figure-part" />
<line x1="140" y1="120" x2="160" y2="100" class="figure-part" />
<!-- legs -->
<line x1="140" y1="150" x2="120" y2="180" class="figure-part" />
<line x1="140" y1="150" x2="160" y2="180" class="figure-part" />
</svg>
<div class="wrong-letters-container">
<div id="wrong-letters"></div>
</div>
<div class="word" id="word"></div>
</div>
<!-- Popup -->
<div class="popup-container" id="popup-container">
<div class="popup">
<h2 id="final-message"></h2>
<h3 id="final-message-reveal-word"></h3>
<button id="play-button">Play Again</button>
</div>
</div>
<!-- Notification -->
<div class="notification-container" id="notification-container">
<p>You have already entered this letter</p>
</div>
Step 2: Styling the Game
The provided CSS code contains styles for various elements in the game. You can modify these styles or add your own to customize the game's appearance. Experiment with colors, fonts, and dimensions to create a visually appealing Hangman game.
1: Global Styles
@import url("https://fonts.googleapis.com/css2?family=DotGothic16&display=swap");: This line imports the "DotGothic16" font from Google Fonts, which is used for the game's text.
2: Body Styles
body: Sets the background color to var(--primary-color), which is a dark blue shade.
color: Sets the text color to var(--light-color), which is a light blue shade.
font-family: Applies the "DotGothic16" font to the body text.
display, flex-direction, align-items, justify-content: These properties set up the flexbox container properties for the body, allowing its contents to be centered both horizontally and vertically.
height, overflow: Sets the height and overflow properties to create a fixed-height container with hidden overflow for the game.
3: Heading Styles
h1: Adds styling to the main heading, including a top margin, letter-spacing, and text transformation.
4: Game Container Styles
padding, position, margin, height, width: Sets the padding, position, margin, height, and width properties for the game container. These values define its dimensions and position on the page.
5: Figure Container Styles
fill, stroke, stroke-width, stroke-linecap: Sets the fill color, stroke color, stroke width, and stroke linecap properties for the figure container SVG element. These properties control the appearance of the hangman figure.
6: Figure Part Styles
display: Hides the figure parts initially using the display: none property.
7: Wrong Letters Container Styles
position, top, right, display, flex-direction, text-align: Sets the position, top, right, display, flex-direction, and text-align properties for the wrong letters container. These properties control its positioning and layout.
8: Wrong Letters Container Paragraph Styles
margin: Sets the bottom margin for the wrong letters container paragraph.
9: Wrong Letters Container Span Styles
font-size: Sets the font size for the wrong letters container spans.
10: Word Styles
display, position, bottom, left, transform: Sets the display, position, bottom, left, and transform properties for the word container. These properties position the word in the center of the game container.
11: Popup Container Styles
background-color, position, top, bottom, left, right, display, align-items, justify-content: Sets the background color, position, top, bottom, left, right, display, align-items, and justify-content properties for the popup container. These properties control its appearance and position on the page.
12: Popup Styles
background-color, border-radius, box-shadow, padding, text-align: Sets the background color, border radius, box shadow, padding, and text-align properties for the popup. These properties style the popup box.
13: Popup Button Styles
cursor, background-color, color, border, margin-top, padding, font-size, font-family, border-radius: Defines the cursor style, background color, text color, border, margin-top, padding, font size, font family, and border radius for the popup buttons. These properties control the appearance of the play again button.
14: Popup Message Styles
font-size, margin-bottom: Sets the font size and margin-bottom properties for the popup message.
15: Notification Styles
position, top, right, background-color, color, border-radius, padding, font-size: Sets the position, top, right, background color, text color, border radius, padding, and font size properties for the notification. These properties control its appearance and position on the page.
@import url("https://fonts.googleapis.com/css2?family=DotGothic16&display=swap");
:root {
--primary-color: #1f2f61;
--secondary-color: #224ca4;
--light-color: #a7c2da;
}
* {
box-sizing: border-box;
}
body {
background-color: var(--primary-color);
color: var(--light-color);
font-family: "DotGothic16", sans-serif;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 80vh;
overflow: hidden;
margin: 0;
}
h1 {
margin: 20px 0 0;
letter-spacing: 0.5rem;
text-transform: uppercase;
}
h2,
h3 {
letter-spacing: 0.2rem;
}
.game-container {
padding: 20px 30px;
position: relative;
margin: auto;
height: 350px;
width: 450px;
}
.figure-container {
fill: transparent;
stroke: var(--light-color);
stroke-width: 4px;
stroke-linecap: round;
}
.figure-part {
display: none;
}
.wrong-letters-container {
position: absolute;
top: 20px;
right: 20px;
display: flex;
flex-direction: column;
text-align: right;
}
.wrong-letters-container p {
margin: 0 0 5px;
}
.wrong-letters-container span {
font-size: 24px;
}
.word {
display: flex;
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
}
.letter {
border-bottom: 3px solid var(--secondary-color);
display: inline-flex;
font-size: 30px;
align-items: center;
justify-content: center;
margin: 0 3px;
height: 50px;
width: 20px;
}
.popup-container {
background-color: rgba(0, 0, 0, 0.3);
position: fixed;
top: 0;
bottom: 0;
left: 0;
right: 0;
display: none;
align-items: center;
justify-content: center;
}
.popup {
background-color: var(--secondary-color);
border-radius: 5px;
box-shadow: 0 15px 10px 3px rgba(0, 0, 0, 0.1);
padding: 20px;
text-align: center;
}
.popup button {
cursor: pointer;
background-color: var(--light-color);
color: var(--secondary-color);
border: 0;
margin-top: 20px;
padding: 12px 20px;
font-size: 16px;
font-family: inherit;
border-radius: 5px;
}
.popup button:active {
transform: scale(0.98);
}
.popup button:focus {
outline: none;
}
.notification-container {
background-color: rgba(0, 0, 0, 0.3);
border-radius: 10px 10px 0 0;
padding: 15px 20px;
position: absolute;
bottom: -60px;
transition: transform 0.3s ease-in-out;
}
.notification-container.show {
transform: translateY(-60px);
}
.notification-container p {
margin: 0;
}
Step 3: Understanding the JavaScript Code
The JavaScript code included in the provided HTML handles the game logic. Let's break down the important parts:
Variables and Arrays:
words: An array containing the words that can be used in the game. You can add or remove words from this array.
selectedWord: A variable that randomly selects a word from the words array for the current game.
playable: A boolean variable that controls whether the game is currently playable or not.
correctLetters: An array to store correctly guessed letters.
wrongLetters: An array to store incorrectly guessed letters.
Functions:
displayWord(): Updates the word display by checking if each letter of the selected word is present in the correctLetters array. It dynamically generates HTML for each letter, making it visible if guessed correctly.
updateWrongLettersElement(): Updates the wrong letters display by appending HTML for each wrong letter in the wrongLetters array.
showNotification(): Displays a notification when the user inputs a duplicate letter.
Event Listeners: The code sets up an event listener for keypress events and handles the logic for processing user input. It checks if the pressed key is a valid letter and updates the correctLetters or wrongLetters array accordingly. It also triggers appropriate functions to update the displays and check for game-ending conditions.
const wordElement = document.getElementById("word");
const wrongLettersElement = document.getElementById("wrong-letters");
const playAgainButton = document.getElementById("play-button");
const popup = document.getElementById("popup-container");
const notification = document.getElementById("notification-container");
const finalMessage = document.getElementById("final-message");
const finalMessageRevealWord = document.getElementById(
"final-message-reveal-word"
);
const figureParts = document.querySelectorAll(".figure-part");
const words = [
"application",
"programming",
"interface",
"wizard",
"element",
"prototype",
"callback",
"undefined",
"arguments",
"settings",
"selector",
"container",
"instance",
"response",
"console",
"constructor",
"token",
"function",
"return",
"length",
"type",
"node",
];
let selectedWord = words[Math.floor(Math.random() * words.length)];
let playable = true;
const correctLetters = [];
const wrongLetters = [];
function displayWord() {
wordElement.innerHTML = `
${selectedWord
.split("") // to array
.map(
(letter) => `
<span class="letter">
${correctLetters.includes(letter) ? letter : ""}
</span>
`
)
.join("")}
`; // to string
const innerWord = wordElement.innerText.replace(/\n/g, "");
if (innerWord === selectedWord) {
finalMessage.innerText = "Congratulations! You won! 😃";
finalMessageRevealWord.innerText = "";
popup.style.display = "flex";
playable = false;
}
}
function updateWrongLettersElement() {
wrongLettersElement.innerHTML = `
${wrongLetters.length > 0 ? "<p>Wrong</p>" : ""}
${wrongLetters.map((letter) => `<span>${letter}</span>`)}
`;
figureParts.forEach((part, index) => {
const errors = wrongLetters.length;
index < errors
? (part.style.display = "block")
: (part.style.display = "none");
});
if (wrongLetters.length === figureParts.length) {
finalMessage.innerText = "Unfortunately you lost. 😕";
finalMessageRevealWord.innerText = `...the word was: ${selectedWord}`;
popup.style.display = "flex";
playable = false;
}
}
function showNotification() {
notification.classList.add("show");
setTimeout(() => {
notification.classList.remove("show");
}, 2000);
}
window.addEventListener("keypress", (e) => {
if (playable) {
const letter = e.key.toLowerCase();
if (letter >= "a" && letter <= "z") {
if (selectedWord.includes(letter)) {
if (!correctLetters.includes(letter)) {
correctLetters.push(letter);
displayWord();
} else {
showNotification();
}
} else {
if (!wrongLetters.includes(letter)) {
wrongLetters.push(letter);
updateWrongLettersElement();
} else {
showNotification();
}
}
}
}
});
playAgainButton.addEventListener("click", () => {
playable = true;
correctLetters.splice(0);
wrongLetters.splice(0);
selectedWord = words[Math.floor(Math.random() * words.length)];
displayWord();
updateWrongLettersElement();
popup.style.display = "none";
});
// Init
displayWord();
Finished Product
Hangman
Find the hidden word - Press a letter
You have already entered this letter
Conclusion
Congratulations! You have successfully created a Hangman game using the provided HTML, CSS, and JavaScript code. By understanding and modifying the code, you can customize the game to your liking and add new features. Hangman is a fun and engaging game that can be enjoyed by players of all ages. Now it's time to challenge your friends and family to a round of Hangman and see who can guess the hidden words correctly!