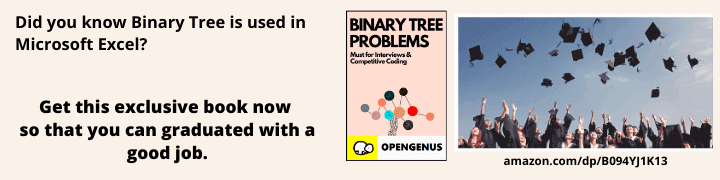
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Flask is a micro web-framework which is easy to learn and is very simple in usage. Yet, it has the capability of building an enterprise level application which can handle large amount of traffic. In this article, we will build basic static webpage application in Flask with no database.
A static webpage means an single HTML code which does not change with user interaction. It may have CSS and JavaScript code linked with it. In terms of performance, this is one of the preferred webpages.
The idea of having no database is to keep the web server lightweight which improves performance significantly. By default, Flask web development framework does not come with a Database (which is not the case with other frameworks like Django).
Setup and Requirements
- Navigate to a convenient directory in your computer.
- Create a new directory called “flaskApp” to start your project.
- You should have code editor or IDE such as Sublime Text, visual studio code, Notepad++
- Install Flask with Pip. In command prompt type $ pip install Flask
Structure
Create the following structure of the project within the “flaskApp” folder in your computer with the following files and folder:
- app.py
- templates
- static
- css
- js
The app.py file will be our Flask aplication file, templates folder will contain HTML files and static folder will contain stylesheets(in css folder), js files(in js folder), images etc.
We will start our application by building a simple html page i.e. static.html which we would be rendering from our Flask application.
static.html
<!DOCTYPE html>
<html>
<head>
<title>My webpage!</title>
<link rel="stylesheet" href="../static/css/style.css" />
<script src="../static/js/index.js" async></script>
</head>
<body>
<h1>Hello, World!</h1>
<h4 id='date'></h4>
<div class="image-section">
<div class="section-style">
<img src="../static/image1.png" alt="" />
<p>Random image 1</p>
</div>
<div class="section-style">
<img src="../static/image2.png" alt="" />
<p>Random image 2</p>
</div>
</div>
<div>
<a href="/static"><button>Click here to go to Dashboard</button></a>
</div>
</body>
</html>
We will also be adding our javascript file in the js folder and name it as index.js.
index.js
document.getElementById('date').innerHTML = new Date().toDateString();
For a bit of styling, we will add css file in css folder in the static folder and name it as style.css
style.css
body {
text-align: center;
background-color: #f0e8c5;
}
.image-section {
display: flex;
justify-content: center;
}
.section-style {
margin-right: 25px;
margin-left: 25px;
background-color: white;
}
div {
margin-top: 15px;
}
We will start building our app.py file by importing neccesary modules.
from flask import Flask, render_template
All Flask web applications must create an application instance that is an object of class Flask which is created as follows:
# create the application object
app = Flask(__name__)
For each url requested, what code would be run is decided by mappings between URLs and the python functions.
This association that handles the mapping in called route.
The most usual way to define a route is through the app.route decorator.
If this application is run on the server associated with the domain www.exampledomain.com, then it would run the function welcome and as a result it would render the home.html file and if we then navigate to http://www.exampledomain.com/dashboard on the browser, it would trigger home function to run. The return value of this home function that is a string "Hello World" is called the response. This response is recieved by the client that is, the web browser.
# use decorators to link the function to a url
@app.route('/')
def welcome():
return render_template('static.html') # render a template
@app.route('/static')
def home():
return "Hello, World!"
The application object (app) has a run method. If we call app.run(), it would launch Flask’s integrated development web server:
# start the server with the 'run()' method
if __name__ == '__main__':
app.run(debug=True)
The name == 'main' ensures that the develop‐
ment server is started only when the script is executed directly.
When the server is started, it goes into loop which takes the requests and services them and the loop is continued until app is stopped.
We can stop the app by hitting Ctrl+C in the terminal.
Our app.py file will look like this:
from flask import Flask, render_template
# create the application object
app = Flask(__name__)
@app.route('/')
def welcome():
return render_template('static.html') # render a template
@app.route('/static')
def home():
return "Hello, World!"
# start the server with the 'run()' method
if __name__ == '__main__':
app.run(debug=True)
We would run out application by writing below code on the terminal.
$ python app.py
Flask vs Django
Flask is a lightweight web application micro-framework. It has been designed to help you get started quickly with web development. Also, it is begginer friendly and has the ability to scale up to complex applications. Now, Flask has become one among the most popular web application frameworks using python.
Flask is convenient for smaller, simply structured and less-complicated projects. Whereas, Django is better suited for handling larger projects.
If you just want a simple web site or RESTful web service that services your mobile application, Flask is better to use than Django.
Question
Flask(__name__) is used for:
With this article at OpenGenus, you must have the complete idea of developing a static webpage application using Flask with no database. Enjoy.