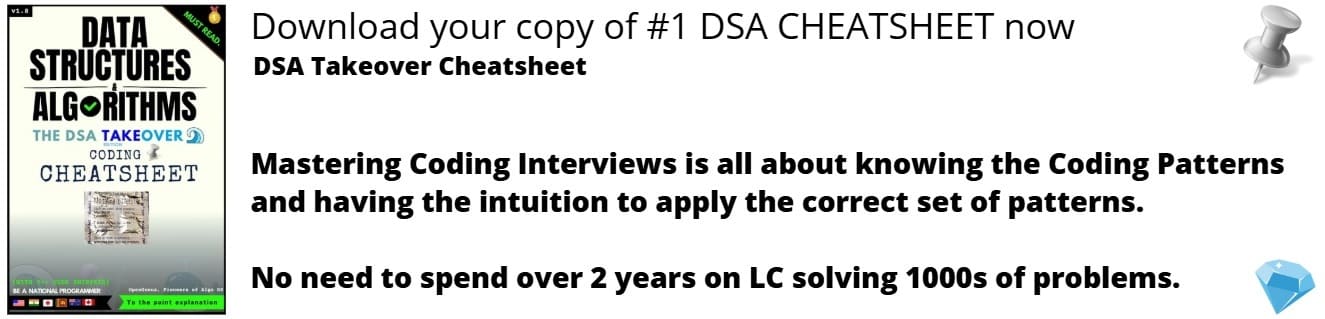
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
TABLE OF CONTENTS:
- Introduction
- Step 1: Create the simple structure of the stopwatch
- Step 2: Create the display to see the time
- Step 3: Create buttons in Stopwatch using HTML CSS
- Step 4: Activate the Stopwatch using JavaScript
Introduction
In this article, we will create a stopwatch/ countdown timer using JavaScript. Basically what we need is a HTML CSS and JavaScript file. The StopWatch uses three buttons which are Start, Pause and Reset. The buttons are used to start, pause and reset the StopWatch. The display will show the progress of the time.
Most informative and useful part of this project is that it can count the hours, minutes, seconds and milliseconds. Firstly we have to make the display then added the buttons and finally make the stopwatch functional.
Step 1: Create the simple structure of the stopwatch
Initial step is create the page and a display box. The purpose of the this box is to display the time. There are three navigation buttons to control the time, the buttons will Pause, Start and Reset the timer. The structure is created using HTML and CSS for the styling. In this project am using deep marron as the background color of the body and white as the background color of the design.
This box will have 40% width and min-width: 500px.
<div class=”container”>
</div>
*,
*:before,
*:after{
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
background: #2b0202;
}
.container{
background-color: #ffffff;
width: 40%;
min-width: 500px;
position: absolute;
transform: translate(-50%,-50%);
top: 50%;
left: 50%;
padding: 20px 0;
padding-bottom: 50px;
border-radius: 10px;
}
Step 2: Create the display to see the time
The next step is to create a display for the timer. We have to create a div
with class timerDisplay
. In this div/display we will be able to count the hours, minutes, seconds, and milliseconds.
The position is relative and a width of 92% and display intiated to flex
. Its given a box-shadow to give it a better user experience
<div class=”timerDisplay”>
00 : 00 : 00 : 000
</div>
.timerDisplay{
position: relative;
width: 92%;
background: #ffffff;
left: 4%;
padding: 40px 0;
font-family: ‘Roboto mono’,monospace;
color: #0381bb;
font-size: 40px;
display: flex;
align-items: center;
justify-content: space-around;
border-radius: 5px;
box-shadow: 0 0 20px rgba(0,139,253,0.25);
}
Step 3: Create buttons in Stopwatch using HTML CSS
In this step we will create the three buttons that are integral to the stopwatch project. The purpose of the is to start, pause and reset the timre. In other words they are set to start the countdown, to pause the timer, and to run the countdown from the beginning again.
I used HTML
button function to create the buttons. Width a stipulated width and height. The background color of the second and third buttons was changed using CSS
code the Nth-last-child
<div class=”buttons”>
<button id=”pauseTimer”>Pause</button>
<button id=”startTimer”>Start</button>
<button id=”resetTimer”>Reset</button>
</div>
.buttons{
width: 90%;
margin: 60px auto 0 auto;
display: flex;
justify-content: space-around;
}
.buttons button{
width: 120px;
height: 45px;
background-color: #d18cf7;
color: #ffffff;
border: none;
font-family: ‘Poppins’,sans-serif;
font-size: 18px;
border-radius: 5px;
cursor: pointer;
outline: none;
}
.buttons button:nth-last-child(2){
background-color: #0a9d1c;
}
.buttons button:nth-last-child(1){
background-color: #d50b0b;
}
Step 4: Activate the Stopwatch using JavaScript
After designing the Stopwatch using HTML and CSS, we need to implement its functionality using JavaScript.
The first step is target a set of information using the JavaScript function called let
. This will a constant of display id and holds 0 for hours, minutes, seconds, and milliseconds.
let [milliseconds,seconds,minutes,hours] = [0,0,0,0];
let timerRef = document.querySelector(‘.timerDisplay’);
let int = null;
let h = hours < 10 ? “0” + hours : hours;
let m = minutes < 10 ? “0” + minutes : minutes;
let s = seconds < 10 ? “0” + seconds : seconds;
let ms = milliseconds < 10 ? “00” + milliseconds : milliseconds < 100 ? “0” + milliseconds : milliseconds;
Next we will execute the start
button using JavaScript. The function document.getElementById
will target the button id and we will another function which is the addEventListener
. The countdown will be initiated when you click this button
document.getElementById(‘startTimer’).addEventListener(‘click’, ()=>{
if(int!==null){
clearInterval(int);
}
int = setInterval(displayTimer,10);
});
The next set of JavaScript function will initialize the pause button upon click
. When the button is clicked, the countdown will pause and a second click will continue the countdown.
document.getElementById(‘pauseTimer’).addEventListener(‘click’, ()=>{
clearInterval(int);
});
The following JavaScript codes will initiate the reset button. The Hours, minutes, seconds, and milliseconds will be back to '0' when you click the reset button.
document.getElementById(‘resetTimer’).addEventListener(‘click’, ()=>{
clearInterval(int);
[milliseconds,seconds,minutes,hours] = [0,0,0,0];
timerRef.innerHTML = ’00 : 00 : 00 : 000 ‘;
});
In this section we will use the function displayTimer
. And also the if
function that when the milliseconds are equal to thousands, the value of milliseconds will be zero and will increase by one in seconds. And when the declared value of milliseconds reaches thousands after the countdown, the value of milliseconds will be zero and the value of seconds will increase by '1'.We will make use of the if
function once agin for the time in seconds. We know one minute is equal to 60 seconds. So when the value of the second is equal to 60, the time of the second will be zero and the minute will increase by '1'.
Since one hour is equal to 60 minutes. When the time in minutes reaches 60, we see 0 in the minute indicator and one increase in the hour.
function displayTimer(){
milliseconds+=10;
if(milliseconds == 1000){
milliseconds = 0;
seconds++;
if(seconds == 60){
seconds = 0;
minutes++;
if(minutes == 60){
minutes = 0;
hours++;
}
}
}
The code was designed to display all this information in the display using innerHTML
. So I stored the hours, minutes, seconds, and milliseconds between h, m, s, and ms, proportionately.
timerRef.innerHTML = ` ${h} : ${m} : ${s} : ${ms}`;
Thanks for reading this article and I hope you have learned how to make a Stopwatch using JavaScript