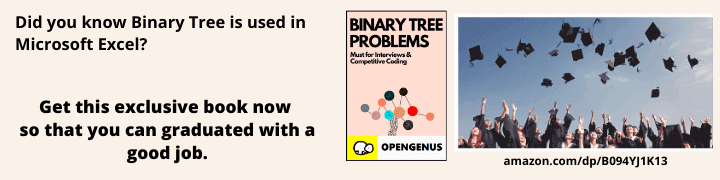
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
The FileReader object helps in reading data from a file or a Blob and store it in a JavaScript variable. The read is done asynchronously so as not to block the browser.
How to define a File Reader object? (Constructor)
The FileReader() constructor creates a new FileReader object. There are no parameters to this constructor.
var reader = new FileReader();
What are the Methods to work on FileReader?
readAsText()
It is used to read the contents of the specified Blob or File. This function returns the file contents as plain text.
var reader = new FileReader();
reader.onload = function(event) {
var contents = event.target.result;
console.log("File contents: " + contents);
};
reader.onerror = function(event) {
console.error("Error faced in reading file : " + event.target.error.code);
};
reader.readAsText(file);
The onload handler is called when the file is successfully read whereas the onerror handler is called if the file wasn’t read for some reason.
We can create a basic webpage to see how this method works. We will try to read an HTML file which has a simple heading line only.
<input type='file' onchange='readFile(event)'><br>
<script>
function readFile(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(event) {
var contents = event.target.result;
console.log("File contents: " + contents);
};
reader.onerror = function(event) {
console.error("Error faced in reading file : " + event.target.error.code);
};
reader.readAsText(file);
}
</script>
Output in console :
File contents: <h2>This is heading.</h2>
readAsArrayBuffer
This function returns the file contents as an ArrayBuffer (can be used for binary data such as images).
<input type='file' onchange='readFile(event)'>
<script>
var readFile = function(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(){
var arrayBuffer = reader.result;
console.log("Size in bytes is " + arrayBuffer.byteLength);
// Output : Size in bytes is 22704
// We opened an image file (22.7 kB)
};
reader.readAsArrayBuffer(file);
};
</script>
readAsDataURL
This method returns the file contents as a data URL. The result attribute contains the data as a data: URL representing the file's data as a base64 encoded string.
<input type='file' onchange='readFile(event)'><br>
<script>
function readFile(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(event) {
var contents = event.target.result;
console.log("File contents: " + contents);
};
reader.onerror = function(event) {
console.error("Error faced in reading file : " + event.target.error.code);
};
reader.readAsDataURL(file);
}
</script>
The output is as follows. We can also decode it using the inbuilt function atob() to see that the contents match.
data:text/html;base64,PGgyPlRoaXMgaXMgaGVhZGluZy48L2gyPgo=
Decode this PGgyPlRoaXMgaXMgaGVhZGluZy48L2gyPgo= to get the contents as original:
<h2>This is heading.</h2>
This method can also be used to display an image that was just read from disk without reloading the full page.
<input type='file' onchange='readFile(event)'><br>
<img id="pic"></img>
<script>
function readFile(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(event) {
var contents = event.target.result;
document.getElementById("pic").src = contents;
};
reader.onerror = function(event) {
console.error("Error faced in reading file : " + event.target.error.code);
};
reader.readAsDataURL(file);
}
</script>
abort
The FileReader.abort() method aborts the read operation. There is also a FileReader.onabort property which contains an event handler, executed when the abort event is fired, i.e. when the process of reading the file is aborted.
What Events are associated with FileReader?
- onloadstart / 'loadstart' - Called after starting a read operation.
- onprogress / 'progress' - Called during a read operation to report the current progress.
- onload / 'load' - Called when a read operation successfully completes.
- onabort/ 'abort' - Called when the read is aborted with abort().
- onerror / 'error' - Called when there is an error during the load.
- onloadend / 'loadend' - Called after a read completes (either successfully or unsuccessfully).
Instance Properties - readyState
It is the current state of the reader - EMPTY, LOADING, or DONE.
- EMPTY (value = 0) - The value returned by readyState before the one of the read methods has been called.
- LOADING (value = 1) - The value returned by readyState after one of the read methods has been called but before the load event has fired.
- DONE (value = 2) - The value returned by readyState after the load event has fired.
<input type='file' onchange='readFile(event)'>
<script>
var readFile = function(event) {
var file = event.target.files[0];
var reader = new FileReader();
reader.onload = function(){
console.log('After load: ' + reader.readyState);
};
console.log('Before read: ' + reader.readyState);
reader.readAsDataURL(file);
console.log('After read: ' + reader.readyState);
};
</script>