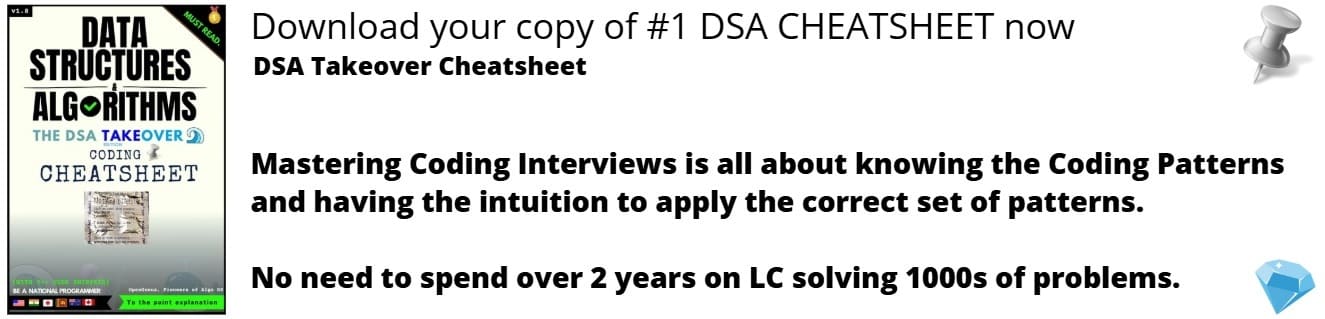
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have listed important Problems on Binary Tree which you must practice for Coding Interviews and listed introductory and background topics on Binary Tree as well. You must bookmark this page and practice all problems listed.
Table of Contents:
- Introduction to Binary Tree + Implementation
- Types of Binary Tree
- Problems on Binary Tree (50+)
(Important)
We have marked the important problems so if you are in a hurry or have limited time, go through the important problems to get the main ideas involving Binary Tree.
Introduction to Binary Tree + Implementation
In this section, we learn about the basics of Binary Tree and how to implement it in different Programming Languages. A very important topic in this section is to implement Binary Tree with no NULL nodes.
- Binary Tree
(Important)
- Properties of Binary Tree
(Important)
- Implementation of Binary Tree in C++
- Implementation of Binary Tree in JavaScript
- Implementation of Binary Tree with no NULL
(Important)
Types of Binary Tree
In this section, we explore Different types of Binary Tree. This enables you to design your own custom Binary Tree and help solve a given problem efficiently.
- Introduction to Skewed Binary Tree
- Threaded Binary Tree
- Binary Search Tree
- Different Self Balancing Binary Trees
(Important)
- AVL Tree
- Splay Tree
(Important)
- 2-3 Tree
- Red Black Tree
- B Tree
- AA Tree
(Important)
- Weight Balanced Binary Tree
- Binary Space Partitioning Tree
(Important)
- Binary Heap
- Binomial Heap
- Treap
Problems on Binary Tree (50+)
"Practice makes you perfect."
You should practice all the Problems listed in this section so that you are comfortable in solving any Coding Problem in an Interview easily. Start by practising 2 problems a day.
These are the different problems on Binary Tree:
- Two Sum Problem in Binary Search Tree: Solved using 3 approaches (DFS, Inorder, Augmented BST)
- Invert / Reverse a Binary Tree: 3 methods: Must read as it uses 3 different approaches (recursive, iterative with stack and queue)
- Types of view in Binary Tree
- Traversing a Binary Tree (Preorder, Postorder, Inorder)
- Convert Inorder+Preorder to Binary Tree (+ other combinations)
(Important)
- Find height or depth of a binary tree
- Find Level of each node from root node
- Diameter of a Binary Tree
- Finding Diameter of a Tree using DFS
- Finding Diameter of a Tree using height of each node
(Important)
- Check if a Binary Tree is Balanced by Height
- Find number of Universal Value subtrees in a Binary Tree
(Important)
- Counting subtrees where nodes sum to a specific value
- Find if a given Binary Tree is a Sub-Tree of another Binary Tree
(Important)
- Check if a Binary Tree has duplicate values
- Find nodes which are at a distance k from root in a Binary Tree
- Finding nodes at distance K from a given node
(Important)
- Find ancestors of a given node in a binary tree
- Largest Independent Set in Binary Tree
- Copy a binary tree where each node has a random pointer
(Important)
- Serialization and Deserialization of Binary Tree
- 0-1 Encoding of Binary Tree
- ZigZag Traversal of Binary Tree
(Important)
- Level order traversal of Binary Tree
- Check if Binary Tree is foldable
(Important)
- Check if 2 Binary Trees are isomorphic
- Convert Binary Tree to Circular Doubly Linked list
- Introduction to Skewed Binary Tree
- Check if Binary Tree is skewed or not
- Change Binary Tree to Skewed Binary Tree
- Threaded Binary Tree
(Important)
- Operations in Threaded Binary Tree
- Convert Binary Tree to Threaded Binary Tree
(Important)
- Binary Search Tree
- Implement Binary Search Tree in C++
- Converting a Sorted Array to Binary Tree
- Minimum number of swaps to convert a binary tree to binary search tree
- Find minimum or maximum element in Binary Search Tree
- Convert Binary Search Tree to Balanced Binary Search Tree
- Find k-th smallest element in Binary Search Tree
- Sum of k smallest elements in Binary Search Tree
(Important)
- Applications of Binary Tree
With this article at OpenGenus, you must have the complete idea of Binary Tree and must be confident in solving any Binary Tree related problem in a Coding Interview instantly. Best of Luck.